Password generation is an important part of every application or website. It is used for generating user credentials or for resetting user passwords. They are also useful for generating strong passwords that users may not be able to come up with on their own. If your application or website is based on python, then you may need to generate random password in python. In this article, we will learn how to create a random password generator in python.
Random Password Generator in Python with Source Code
We will create a simple password generator that asks users for number of characters to be used for passwords, and then generate a random password consisting of alphabets, digits & special characters accordingly.
1. Import Required Modules
First, create a blank python file.
$ vi password_generator.py
Add the following lines to import required modules into it. We will use random and string modules for our code.
import string import random
2. Create Character List
Next, we will create a python list of characters to be used in passwords. It will consist of all digits, alphabets & some special characters. So add the following lines to your python script.
characters = list(string.ascii_letters + string.digits + "!@#$%^&*()")
3. Define Password Generator Function
We will define a function generate_password() that will contain the code for password generation. Here is the code for it. We will look at it in detail.
def generate_random_password(): ## length of password from the user length = int(input("Enter password length: ")) ## shuffling the characters random.shuffle(characters) ## picking random characters from the list password = [] for i in range(length): password.append(random.choice(characters)) ## shuffling the resultant password random.shuffle(password) ## converting the list to string ## printing the list print("".join(password))
In the above code, we use input() function to prompt the user to enter the number of characters to be used in password. The user input is stored in variable length.
We call random.shuffle() function to shuffle the characters in out list of characters. Next, we create an empty list to store password characters.
Then we create a for loop with number of iterations equal to the password length specified by user. In each iteration of for loop, we use random.choice() function to pick a random character from our list of characters.
After for loop terminates, we call random.shuffle() function on the password python list. This is optional and can be skipped if you want.
Finally, we call join() function to concatenate the characters present in password list to form our password string.
You can call the password generator function as shown below.
generate_password()
Here is the complete code for your reference.
import string import random characters = list(string.ascii_letters + string.digits + "!@#$%^&*()") def generate_random_password(): ## length of password from the user length = int(input("Enter password length: ")) ## shuffling the characters random.shuffle(characters) ## picking random characters from the list password = [] for i in range(length): password.append(random.choice(characters)) ## shuffling the resultant password random.shuffle(password) ## converting the list to string ## printing the list print("".join(password)) generate_password()
4. Make Script Executable
Run the following command to make it executable.
$ sudo chmod +x password_generator.py
You can run the python script using the following command.
$ python password_generator.py
In this article, we have learnt how to create password generator using python. You can customize it as per your requirement. For example, you can even modify the character list to consist of only digits, fix the input length and modify the code into an OTP (one time password) generator, used for financial transactions and authentication. Generally, these password generators are a part of larger modules and applications, so you can add it to your application as you need it.
Also read:
Generator Random Password in Linux
How to Use Wget to Download Files via Proxy
How to Get Hostname/Domain Name from IP Address
How to Check if String Matches Regular Expression
How to Modify XML File in Python
Related posts:
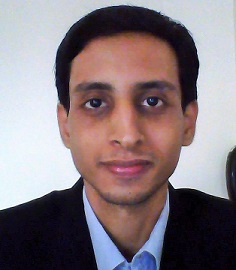
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.