Python is a powerful programming language that supports many different types of data including bytes. In most cases, you can directly use byte-type data as strings. But if you are getting an error on your system, then you may need to explicitly convert bytes into string in Python. In this article, we will look at different ways to convert bytes to string in python.
How to Convert Bytes to String in Python
By default, you should be able to directly use byte data as strings. Here is an example on python 2.7.3 where we declare a byte variable and use it as a string.
>>> a=b"abcde" >>> a 'abcde' >>> str(a) 'abcde' >>> type(a) <type 'str'>
If the above code doesn’t work on your system, then you can explicitly convert bytes to string using decode() function.
On Python 2
>>> b"abcde".decode("utf-8") u'abcde'
Alternatively, you can also use unicode() function for the same purpose.
>>> unicode('hello', 'utf-8') u'hello'
On Python 3
You can also use decode() function in Python 3.
>>> encoding = 'utf-8' >>> b'hello'.decode(encoding) u'hello' OR >>> str(b'hello', encoding) u'hello'
In this short article, we have learnt how to convert bytes into strings. You can use these codes in your python script or application, if you are getting errors while trying to use bytes or bytearray data-type as strings.
Also read:
How to Store Output of Cut Command to Shell Variable
How to Use Shell Variables in Awk Script
How to Setup SSH Keys in Linux
How to Create Nested Directory in Python
How to Find Package for File in Ubuntu
Related posts:
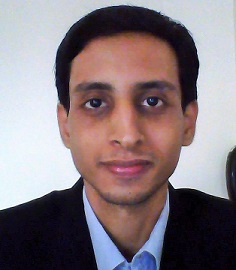
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.