Python offers many modules & functions to help you work with files. But while working with files, it is essential to first check if the file exists, before you work with them. Otherwise, python will throw a FileNotFoundError Exception. Although you may use try…catch method to catch the errors, it is cumbersome to implement and understand when a file does or does not exist. It is advisable to check if file exists without throwing an exception. In this article, we will learn how to check if file exists in Python.
How to Check if File Exists in Python
We will look at a couple of ways to check if a file exists in python – using os and using pathlib.
1. Using os
First import os.path library.
import os.path
Next call the path to file using exists() function.
os.path.exists(path_to_file)
You may specify relative path or full path. If you specify relative path then python determine its path relative to its current working directory during runtime. If you specify only the filename then python will try to find it in the current working directory.
Also, it is advisable to use front slash in path such as /home/ubuntu whether you are using Windows, Linux or Mac.
Here is a simple code to determine if a file exists or not.
import os.path file_exists = os.path.exists('data.txt') print(file_exists)
If the file exists, the output will be True, else it will be False.
2. Using pathlib module
You can also use pathlib module, if you are using python >=3.4. It allows you to work with files and folders as objects.
First, import pathlib module and Path class from it.
from pathlib import Path
Next, use the Path function to instantiate an object for file path.
path = Path(path_to_file)
Next, use is_file() function to determine if the path exists or not.
path.is_file()
Here is a simple example code using pathlib.
from pathlib import Path path_to_file = 'data.txt' path = Path(path_to_file) print(path.is_file())
In the above code, if the file exists, then python will print True, else it will print False. Using these values, you can appropriately process the file.
In this article, we have seen two ways to check if file exists in Python. You can use these functions in your code to ensure that python does not throw an exception if the file does not exist at specified path.
Also read:
How to Allow MySQL User from Multiple Hosts
How to Check Python Package Dependencies
How to Check Python Package Path
How to Take Screenshot in Ubuntu Terminal
How to Make Cross Database Queries in PostgreSQL
Related posts:
How to Import Python Modules by String Name
How to Export Pandas Dataframe to Excel
How to Split String With Multiple Delimiters in Python
How to Count Repeated Characters in String in Python
How to Write List to File in Python
How to Select Multiple Columns in Pandas Dataframe
How to Convert PDF to CSV in Python
How to Check if String is Integer in Python
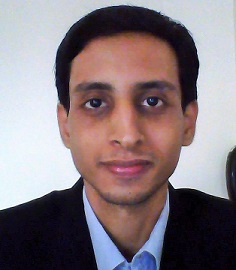
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.