Python Pandas is a library that allows you to easily work with data, import & export them to & from files respectively. It supports a wide range of functions to transform and process your data. Often you may need to export Pandas dataframe to Excel to be able to analyze it further in spreadsheets, or share them with others. In this article, we will learn how to export Pandas Dataframe to Excel.
How to Export Pandas Dataframe to Excel
Here are the steps to export Pandas Dataframe to Excel. Dataframe is the central object to almost all processing in Pandas. When you import data from file, you typically store in a Pandas Dataframe object. When you perform transformations on it, it still remains within the dataframe object. So many times you may need export it in another format, in order to be able to access data outside python. Python pandas library provides the useful to_excel() function to easily write a dataframe to an excel document. We will see a couple of ways to write Python Pandas dataframe to excel spreadsheet.
1. Directly Export to Excel
In this case, we directly call to_excel() function to export data. Here is an example where we import Python pandas module, create a dataframe, and then call to_excel() function to export the dataframe to excel document.
# importing the module import pandas as pd # creating the DataFrame cars_data = pd.DataFrame({'Cars': ['BMW', 'Audi', 'Bugatti', 'Porsche', 'Volkswagen'], 'MaxSpeed': [220, 230, 240, 210, 190], 'Color': ['Black', 'Red', 'Blue', 'Violet', 'White']}) # determining the name of the file file_name = 'Data.xlsx' # saving the excel cars_data.to_excel(file_name) print('DataFrame is written to Excel File successfully.')
2. Using ExcelWriter()
You can alternatively use ExcelWriter() method on the Excel spreadsheet.
# importing the module import pandas as pd # creating the DataFrame cars_data = pd.DataFrame({'Cars': ['BMW', 'Audi', 'Bugatti', 'Porsche', 'Volkswagen'], 'MaxSpeed': [220, 230, 240, 210, 190], 'Color': ['Black', 'Red', 'Blue', 'Violet', 'White']}) # writing to Excel datatoexcel = pd.ExcelWriter('Data.xlsx') # write DataFrame to excel cars_data.to_excel(datatoexcel) # save the excel datatoexcel.save() print('DataFrame is written to Excel File successfully.')
In this article, we have learnt how to export pandas dataframe to excel. You can customize this code as per your requirement.
Also read:
How to Export Pandas Dataframe to PDF
How to Run Python Script in Apache Web Server
Shell Script to Delete/Clear Log Files
How to Exclude Requests from Apache Log
How to Exclude Requests from NGINX Log
Related posts:
How to Merge Folders & Directories in Python
How to Get Difference of Two Dictionaries in Python
How to Convert Columns into Rows in Pandas
How to Pad String in Python
How to Import from Parent Folder in Python
How to Convert All Strings in List to Integer
How to Stop Python Code After Certain Amount of Time
How to Execute Stored Procedure in Python
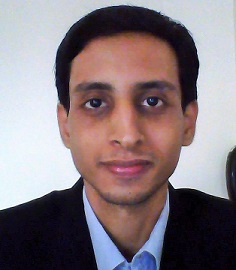
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.