Lists are versatile data structures used by python developers for almost every application and website. They provide tons of features and functions to help you perform various tasks. Sometimes you may need to write list to file in Python. In this article, we will learn how to do this.
How to Write List to File in Python
Let us say you have the following list in python.
lines = ['hello', 'world', 'good', 'morning']
Let us say you want to write its contents into a file /home/ubuntu/data.txt one list item on each line followed by newline character. You can easily do this using the following code snippet.
with open('/home/ubuntu/data.txt', 'w') as f: for line in lines: f.write(f"{line}\n")
In the above code, we open the file data.txt for writing. Then we run a for loop through our list, one item at a time. In each iteration, we write the item to file using file.write() command. In this function, we also append newline character ‘\n’ after the line item. You can remove it if you don’t want to add a newline character after every line.
If you are using python < 3.6 you may need to modify the above code as shown below.
with open('/home/ubuntu/data.txt', 'w') as f: for line in lines: f.write("%s\n" % line)
If you are using python 2, you can also use the following code snippet.
with open('/home/ubuntu/data.txt', 'w') as f: for line in lines: print >> f, line
If the above codes do not work for you, try the following code. It should work for all python > 2.6
with open('/home/ubuntu/data.txt', 'w') as f: for item in lines: f.write("{}\n".format(item))
In all above code snippets, make sure to use the full path to your file so that you don’t get a ‘file not found’ error.
There are several ways to write list to file in python. You can use any of the above methods as per your requirement.
Also read:
How to Fix Invalid Use of Group Function in MySQL
How to Insert Current Datetime in PostgreSQL
How to Insert Current Datetime in MySQL
How to Uninstall MySQL Completely in Ubuntu
How to View MySQL Query Locking Table
Related posts:
How to Check Version of Python Modules
How to Comment in Python
How to Convert Webpage into PDF using Python
How to Check if Key Exists in Python Dictionary
How to Create Nested Directory in Python
How to Get Classname of Instance in Python
How to Import from Another Folder in Python
How to Sort List of Tuples by Second Element in Python
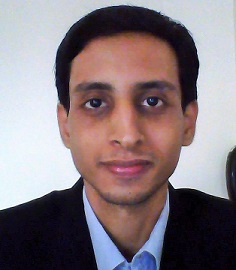
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.