IFrame is often used to display content of other web pages and websites on a given web page. But often, you may find that this content doesn’t fit the size of IFrame or is too small for it. In such cases, if you do not resize IFrame based on content it might look awkward for your users. In this article, we will learn how to auto resize IFrame based on content using JavaScript. Using this you don’t have to worry about an IFrame’s content not fitting its dimensions.
How to Auto Resize IFrame Based on Content
Let us say you have the following IFrame.
<iframe src="/test.html" id="myFrame"></iframe>
Now IFrame will be displayed as per its CSS whereas the /test.html will be displayed as per its own styling irrespective of the size of IFrame. To fix this problem, you can write a JS function that causes the IFrame to resize on its own once its content is loaded. Here is the code snippet for it.
<script type="application/javascript"> function resize_iframe( iFrame ) { iFrame.width = iFrame.contentWindow.document.body.scrollWidth; iFrame.height = iFrame.contentWindow.document.body.scrollHeight; } window.addEventListener('DOMContentLoaded', function(e) { var iFrame = document.getElementById( 'myFrame' ); resize_iframe( iFrame ); } ); </script>
In the above code, we first define resize_iframe() function that sets the width and height of IFrame by calling contentWindow.document.body.scrollWidth and contentWindow.document.body.scrollHeight properties respectively.
Then we add an event listener to Window for the event DOMContentLoaded. It is called when the IFrame content is loaded.
Now when the IFrame content is loaded, resize_iframe() function is called and it is auto resized based on the dimensions of the content.
If you have multiple IFrames on your page, then you can modify the above code to loop through each IFrame and call resize_iframe() function on each IFrame.
var iframes = document.querySelectorAll("iframe"); for( var i = 0; i < iframes.length; i++) { resize_iframe( iframes[i] ); }
In this article, we have learnt how to auto resize iFrame using JavaScript. You can customize it as per your requirement.
Also read:
How to Count Substring Occurrence in JS String
How to Convert Comma Separated String into JS Array
How to Suppress Warning Messages in MySQL
How to Show JavaScript Date as AM/PM Format
How to Get HTML Element’s Actual Width & Height
Related posts:
How to Clear HTML5 Canvas for Redrawing in JavaScript
How to Clone Array in JavaScript
How to Calculate Age from DOB in JavaScript
How to Check if Variable is Undefined in JavaScript
How to Find DOM Element Using Attribute Value
How to Create Please Wait Loading Animation in jQuery
How to Set/Unset Cookie With jQuery
How to Get Array Intersection in JavaScript
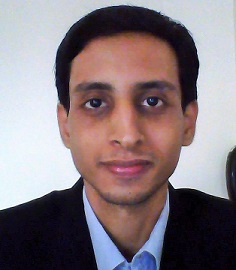
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.