Sometimes you may need to get HTML element’s actual width & height. There are several ways to do this in JavaScript as well as third party libraries like jQuery. In this article, we will learn how to do this the right way.
How to Get HTML Element’s Actual Width & Height
Typically, people use offsetWidth and offsetHeight to get the width and height of DOM element respectively. For example, if you want to get height and width of div with id=myDiv, then here is how most people retrieve it.
var width = document.getElementById('myDiv').offsetWidth; var height = document.getElementById('myDiv').offsetHeight;
But the problem with this approach is that offsetWidth and offsetHeight are properties belonging to the element and not its style. So in some cases they may return value of 0 if you have modified the DOM element.
So it is advisable to use getBoundingClientRect() function to obtain a DOM element’s width and height. It returns the dimensions and location of DOM element as floating point numbers after performing CSS transformations. Here is an example of what its output looks like.
console.log(document.getElementById('myDiv').getBoundingClientRect()) DOMRect { bottom: 187, height: 57.7, left: 218.5, right: 809.5, top: 112.3, width: 531, x: 218.5, y: 112.3, }
So you can easily get the correct values for height and weight of DOM element as shown below.
var positionInfo = document.getElementById('myDiv').getBoundingClientRect(); var height = positionInfo.height; var width = positionInfo.width;
If you use a third-party library like jQuery, you can use its built-in height() and width() functions as shown below.
var height = $("#myDiv").height(); var width = $("#myDiv").width();
In this article, we have learnt a couple of ways to get height and width of DOM element in JavaScript and jQuery.
Also read:
How to Call Parent Window Function from IFrame
How to Get Cookie By Name in JavaScript
How to Find Max Value of Attribute in Array of JS Objects
How to Get Last Item in JS Array
How to Check if Variable is Object in JS
Related posts:
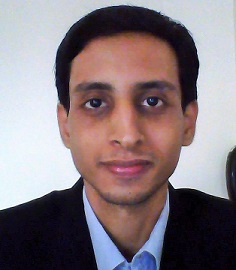
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.