JavaScript supports numerous different types of data structures to store data. It also supports JS objects that allow you to store information as key-value pairs, in a compact manner. They are similar to JSON format and are popularly used to exchange data with web servers, by converting them to JSON format. However, if you try to access a JS object that does not exist, or a variable that is not a JS object, then you may get an error and your JS code will stop working. So it is advisable to check if variable is object in JS before using it. In this article, we will learn how to do this.
How to Check if Variable is Object in JS
There are plenty of different ways to check if variable is object in JS. In fact, there are even third party libraries for this purpose. But we will go with the simplest way to do this using typeof operator that is natively supported by JavaScript in all modern browsers.
Here is the syntax of typeof operator.
typeof variable
Depending on the data type of variable, typeof operator returns string, number, boolean, object, function, null, undefined. So if your variable is an object, then typeof operator will return ‘object’ else it will return one of the other values. You can use this with if condition to check if variable is object or not.
if(typeof variable === 'object'){ console.log('variable is object'); }else{ console.log('variable is not object'); }
If you want to exclude certain data types from above check, you can modify your code as shown below. It excludes null, array and functions.
if ( typeof yourVariable === 'object' && !Array.isArray(yourVariable) && yourVariable !== null ) { console.log('variable is object'); }
In this article, we have learnt an easy way to check if variable is object or not. You can use the same method to check if variable is any other type of data, as per your requirement.
Also read:
How to Check if Browser Tab is Active
How to Find DOM Element Using Attribute Value
How to Generate Hash from String in JavaScript
How to List Properties in JS Object
How to Detect if Device is iOS
Related posts:
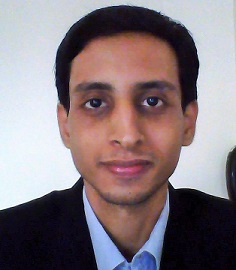
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.