If you are trying to make your website visitors stay on your website or application then you need to make sure that the browser tab that is displaying your web page is active as much as possible. Otherwise, users may minimize their web browser or switch to another tab and move on to other tasks. In this article, we will learn how to check if browser tab is active or not.
How to Check if Browser Tab is Active
There are several ways to check if browser tab is active or not. We will look at some of the simple ones
1. Using JavaScript
You can use the document.hidden property to check if browser tab is active or not. If a web browser tab is active then its document.hidden property is false, else it is true.
if (!document.hidden) { // do what you need }
If you want to periodically check whether your browser tab is active or not, you can run the above code from within setInterval() function as shown below. The following example checks if browser tab is active or not, every 5 seconds.
setInterval(function () {if (!document.hidden) { // do what you need }}, 5000);
Alternatively, you can also use Page Visibility API for this purpose. However it only checks if web browser has been minimized or covered by another window. It does not check if browser tab is active or not.
Sometimes you may need to use a combination of both to see if your browser is not minimized, as well as your tab is active.
Another way to check whether web browser is active or not is given below.
var isTabActive; window.onfocus = function () { isTabActive = true; }; window.onblur = function () { isTabActive = false; }; setInterval(function () { console.log(window.isTabActive ? 'active' : 'inactive'); }, 1000);
In the above code, we attach event handlers for window.onfocus and window.onblur events. window.onfocus is called when browser tab is active, window.blur is called when it is minimized or covered by other windows. When window is active, we set isTabActive to true, else we set it to false. We also check this value every 1 second, using setInterval() function to determine if the browser tab is active or not.
2. Using jQuery
jQuery also makes it easy to check if web browser is active or not. Here is a sample code snippet for this purpose.
var interval_id; $(window).focus(function() { if (!interval_id) interval_id = setInterval({/*do your work here*/}, 1000); }); $(window).blur(function() { clearInterval(interval_id); interval_id = 0; });
In the above code, we define event handlers for window.focus and window.blur events. window.blur event will be called when your browser is minimized or covered by another window. window.focus is called when user is viewing your browser.
When your web browser is active, window.focus() is called and it regularly runs the setInterval() function every 1 second. When user minimizes browser window or it goes out of focus, window.blur() function is called and it clears the timeout function by calling clearInterval().
In this article, we have learnt a couple of easy ways to check if web browser is active or not. You can customize them as per your requirement.
Also read:
How to Find DOM element using attribute value
How to Generate Hash from String in JavaScript
How to List Properties of JS Object
How to Detect if Device is iOS
How to Count Character Occurrence in JS String
Related posts:
How to Remove Empty Element from JS Array
How to Access Iframe Content With JavaScript
How to Bind Event to Dynamic Element in jQuery
How to Check if JS Object Has Property
How to Select Element By Text in JavaScript
How to Escape HTML Strings With jQuery
How to Attach Event to Dynamic Elements in JavaScript
How to Preload Images with jQuery
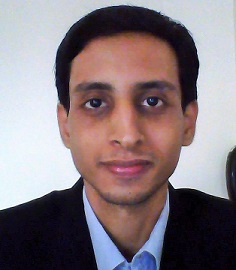
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.