JavaScript allows you to select DOM elements in numerous ways using ID, tag, name, class, attributes, etc. Sometimes you may need to select DOM elements based on their HTML text, in JavaScript. In this article, we will learn how to select element by text in JavaScript.
How to Select Element By Text in JavaScript
Let us say you have the following HTML element.
<a ...>SampleText</a>
There are several ways to select element by text in JavaScript.
1. Using XPath
XPath is a simple way to search an element using query selectors.
var xpath = "//a[text()='SampleText']"; var matchingElement = document.evaluate(xpath, document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue;
2. Using DocumentElement
You can also use outerHTML and innerHTML properties to select DOM elements using HTML text. Here is an example to select elements based on outerHTML attribute.
document.documentElement.outerHTML.includes('SampleText')
Similarly, you can also select DOM elements using innerHTML property.
document.documentElement.innerHTML.includes('SampleText')
3. Using JQuery
JQuery is a powerful and popular JavaScript library that offers many useful functions. You can use the contains() function to easily select DOM elements using their innerHTML text.
var element = $( "a:contains('SampleText')" );
In this article, we have learnt how to select DOM elements using their text values.
Also read:
How to Select Element By Name in JavaScript
How to Get Value of Selected Radio Button
How to Disable Browser AutoFill Using JavaScript
How to Stop Browser Back Button Using JavaScript
How to Disable Clicks in IFrame Using JavaScript
Related posts:
How to Check if Variable is Undefined in JavaScript
How to Split Array Into Chunks in JavaScript
How to List Properties of JS Object
How to Get Unique Values from Array in JavaScript
How to Check if Variable is Object in JS
How to Get data-id attribute in jQuery / JavaScript
How to Count Character Occurrence in String in JS
How to Check if String Starts With Another String in JavaScript
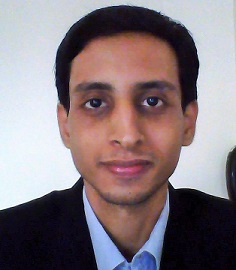
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.