JavaScript objects are powerful data structures that allow you to easily store different data types in a compact manner. Sometimes you may need to check if JS object is array in JavaScript. There are several ways to do this in JavaScript. In this article, we will learn how to check if object is array.
How to Check if Object is Array in JavaScript
Let us say you have the following JS object.
test = {'a':1, 'b':2}
Here are some of the ways to check if JS object is array in JavaScript.
1. Using Constructor
Every JS variable has constructor property available by default. You can simply use the constructor property of JS object to check if it is an array or not, as shown below. If it is an array then the constructor’s value will be Array.
test.constructor === Array OR test.constructor.name === "Array"
If you face issues with above command, first you can check if the constructor property is available or not.
test.prop && test.prop.constructor === Array
2. Using InstanceOf
You can also use InstanceOf keyword to check if variable is array or not.
test instanceof Array
3. Using Object.prototype
This is most complicated but also works for checking any data type not just arrays. The above two methods work only for checking if a variable is Array or not. You can use this method to check if a variable is string, or some other data type, just by changing [object Array] below to data type of your choice.
if(Object.prototype.toString.call(test) === '[object Array]') { alert('Array!'); }
In this article, we have learnt a few simple ways to check if a JS object is array or not. You can also use these methods to check if any variable, not just JS object, is array.
Also read:
Regular Expression to Match URL Path
How to Split Every Nth Character in Python
How to Reverse/Invert Dictionary Mapping
How to Sort List of Tuples by Second Element in Python
How to Get Key With Max Value in Dictionary
Related posts:
How to Validate Decimal Number in JavaScript
Remove Unicode Characters from String
How to Split Array Into Chunks in JavaScript
How to Use Dynamic Variable Names in JavaScript
How to Close Current Tab in Browser Window
How to Prevent Web Page from Being Loaded in Iframe
How to Generate Random Color in JavaScript
How to Add Days to Date in JavaScript
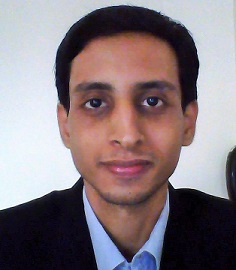
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.