Python is a powerful programming language used by many software developers. It provides plenty of useful features to help you quickly build applications & websites. It also allows you to easily work with files and folders. Sometimes you may need to remove file or folder from your system, using python. In this article, we will learn the steps to delete file or folder using Python.
How to Delete File or Folder in Python
There are several ways to delete file or folder in Python.
1. Using os
os is a built-in module in every python and it allows you to work with operating system & disks. os module provides remove() function to delete files and rmdir() function to delete folders. Here are the commands to delete file /data/test.txt
import os #remove file os.remove('/data/test.txt')
os.remove() function requires you to provide file path. If you don’t provide full path but only relative path, then python will look for the file in its current working directory.
If the path provided turns out to be a directory, it will throw IsADirectoryError error. If the file does not exist, then it throws FileNotFoundError error.
Here is the command to delete folder /data/projects
import os #remove folder os.rmdir('/data/projects')
Here too, if the folder does not exist, then it will throw FileNotFoundError error. If the directory is not empty, then it will throw OSError error.
If you want to delete a directory recursively, use shutil module as shown below.
2. Using Shutil
Shutil is another standard library offered by python that allows you to easily work with files and folders. rmtree() function is one such utility. Here is the command to recursively delete a folder /data/projects and all its contents.
import shutil #recursively delete folder shutil.rmtree('/data/project')
In the above command, it is advisable to specify the full path of folder. If you specify only relative path, then python will look for the file in its present working directory.
3. Using Path module
From python 3.4, you can also use path module to delete files and folders.
import pathlib #remove file pathlib.Path.unlink('/data/test.txt') #remove folder pathlib.Path.rmdir('/data/project')
Here too, it is important to remember to provide full path to file or folder, in order to avoid ‘File Not Found’ errors. Also please ensure that the folder is empty before you use rmdir() function. Otherwise, you will get an error.
Since these functions give an error if the file does not exist or if the folder is not empty, it is advisable to check these things first, before you proceed with the deletion. Here is a simple example to check if the file or folder exists, before deleting it.
if os.path.isfile('/data/test.txt'): os.remove('/data/test.txt')
Alternatively, you can also wrap the code for file/folder deletion in try-catch block.
try: os.remove('/data/test.txt') catch: #handle error
In this article, we have looked at several ways to delete files & folders in Python. There are also several third-party tools to work with files & folders but the built-in standard libraries like os and shutil are sufficient for deletion or files & folders.
Also read:
How to Disable Django Error Emails
How to Add Minutes, Hours & Months to Datetime in PostgreSQL
Find Files with Special Characters in Filename in Linux
Find Last Occurrence of Character in String in JS
Find Last Occurrence of Character in String in Python
Related posts:
How to Import from Parent Folder in Python
How to Compare Strings in Python
How to Check if File Exists in Python
How to Extract Tables from PDF in Python
How to Merge Folders & Directories in Python
How to Concatenate Multiple Lists in Python
How to Create Python Dictionary from String
How to Run Python Script in Django Project
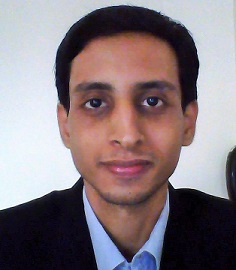
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.