Python is a powerful language that allows you to work with many data types including strings. Sometimes you may need to find last occurrence of substring or character in string in Python. There are a couple of simple ways to do this. In this article, we will learn how to find last occurrence of character in string in Python.
How to Find Last Occurrence of Character in String in Python
You can find last occurrence of character in string in python using rfind() and rindex() functions. We will look at each of these functions one by one.
1. Using rindex()
rindex() function finds the last occurrence of character or string and returns the index of the starting position of substring. If there is no such character or substring, then rindex() will return an exception.
Here is the syntax of rindex().
string.rindex(value, start, end)
In the above command, you need to specify the character or substring whose last occurrence you want to find, followed by optional arguments of starting & ending position of original string, within which you want to find the character or substring. By default, the starting position is 0 and ending position is the last character of the string.
Let us look at a few examples.
Here is an example to find the last occurrence of ‘in’ in our string.
>>> a='hello tin tin. good morning' >>> a.rindex('in') 24
Here is an example that throws an exception when character or substring is not found.
>>> a.rindex('out') Traceback (most recent call last): File "<pyshell#3>", line 1, in <module> a.rindex('out') ValueError: substring not found
Here is an example to find last occurrence of single character using rindex().
>>> a.rindex('n') 25
2. Using rfind()
rfind() is similar to rindex() in terms of syntax and functionality. The main difference between rindex() and rfind() is that rindex() throws an exception if it is unable to find the specified character or substring while rfind() returns -1.
Here is the syntax of rindex().
string.rfind(value, start, end)
In the above command, you need to specify the character or substring whose last occurrence you want to find, followed by optional arguments of starting & ending position of original string, within which you want to find the character or substring. By default, the starting position is 0 and ending position is the last character of the string.
Let us look at a few examples.
Here is an example to find the last occurrence of ‘in’ in our string.
>>> a='hello tin tin. good morning' >>> a.rfind('in') 24
Here is an example that throws an exception when character or substring is not found.
>>> a.rfind('out') -1
Here is an example to find last occurrence of single character using rindex().
>>> a.rfind('n') 25
That’s it. In this article, we have learnt two simple ways to find the last occurrence of character or string in python. If you want python to throw an error in case the specified character or substring is not found, use rindex(), else use rfind().
Also read:
How to Split Python List into N Sublists
How to Insert Text At Certain Line in Linux
Django Get Unique Values from Queryset
How to Create RPM for Python Module
What is NoReverseMatch Error & How to Fix it
Related posts:
Python Script to Check URL Status
What Is __name__ In Python
How to Convert Webpage into PDF using Python
How to List All Virtual Environments in Python
How to Merge PDF Files Using Python
How to Run Python Script in Apache Web Server
How to Iterate Through List of Dictionaries in Python
How to Password Protect PDF in Python
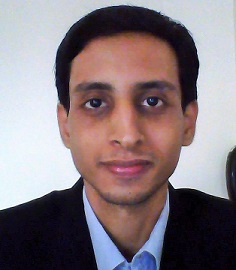
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.