Python Lists are powerful data structures that help you store diverse data types and work with them. Sometimes you may need to split python list into N sublists. In this article, we will learn how to split python list into sublists using python’s numpy library which offers many features & functions to work with python data types.
How to Split Python List into N Sublists
We will use python numpy to split lists. If your python does not have numpy yet, open terminal and run the following command to install it via pip.
$ pip install numpy
If you don’t have pip either, here are the steps to install it on your Linux system.
Please note, numpy will split list such that all sublists will have equal number of items, as far as possible. If that is not possible, then some of the sublists will have one or more elements than the others.
When we use numpy to split lists, the output will be an array of N lists. Here is an example to split an array into 2 parts using split function.
>>> import numpy as np >>> mylist = np.array([1,2,3,4,5,6]) >>> np.split(mylist, 2) [array([1, 2, 3]), array([4, 5, 6])]
You can save the output to a variable in order to use it further.
>>> output=np.split(mylist, 2) >>> output[0] array([1, 2, 3])
You can also split the list using array_split function.
>>> np.array_split(mylist, 2) [array([1, 2, 3]), array([4, 5, 6])]
In the above example, you can see that the 2 lists have been equally divided. But what if it is not possible to split list items equally among sublists?
In such cases, if you use split() function, you will get an error, and if you use array_split() function, then some lists will have more items than the others. Let us try to split the above list into 4 parts using split() function first, and then using array_split()
>>> np.split(mylist,4) Traceback (most recent call last): File "<pyshell#9>", line 1, in <module> np.split(mylist,4) File "C:\Python27\lib\site-packages\numpy\lib\shape_base.py", line 849, in split 'array split does not result in an equal division') ValueError: array split does not result in an equal division >>> np.array_split(mylist,4) [array([1, 2]), array([3, 4]), array([5]), array([6])]
As you can see, array_split() function splits the list into unequal lists but does not give an error.
So if you don’t want to get an error message but want to split list into sublists, even if they are unequal in size, then use array_split. On the other hand, if you don’t want python to split a list into sublists with unequal items but want to throw an error, then use split() functions.
Of course, there are many ways to split a list into sublists, and we have shown a very easy to ways to do this.
Also read:
How to Insert Text at Certain Line in Linux
Django Get Unique Values from Queryset
How to Create RPM for Python Module
What is NotReverseMatch Error & How to Fix It
How to Create RPM for Script
Related posts:
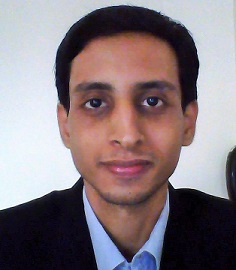
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.