Sometimes you may need to delete all instances of character from string in Python. In this article, we will learn how to delete all instances of character from string in Python.
How to Delete All Instances of Character from String
Python provides a simple function called replace() to replace all occurrences of character or substring in a string, in python. Here is its syntax.
str.replace(old_substring, new_substring)
Replace function can be called on every string in Python. You need to specify the substring to be replaced, as the first argument, and the new string as the second argument.
In order to delete all occurrences of character in a string, we will use the character as first argument, and use empty quotes (” or “”) as the second argument. Here is an example to replace all occurrences of ‘l’ in string ‘hello world’.
a='hello world' a.replace('l','') 'heo word'
Please note, the replace function only replaces the given substring in the output but leaves the original strings unchanged, as shown.
a='hello world' a.replace('l','') 'heo word' print(a) 'hello world'
So if you want to save the new string or re-use it, you need to assign it to a variable.
a='hello world' b=a.replace('l','') print(b) 'heo word'
Or you can re-assign the replaced string to the original string, as shown below.
a='hello world' a=a.replace('l','') print(a) 'heo word'
In this short article, we have learnt how to remove all occurrences of a character in a string, in python. You can use the same trick to delete all occurrences of substring, not just character.
On the other hand, if you want to remove only the first occurrence of character then add 1 as the third argument in replace() function, as shown below.
>>> a='hello world' >>> a 'hello world' # replace first occurrences >>> a.replace('l','',1) 'helo world' # replace first 2 occurrences >>> a.replace('l','',2) 'heo world'
Also read:
How to Randomly Select Item from List in Python
How to Concatenate Strings in MySQL
What is Basename Command in Shell Script
How to Compare Strings in Python
How to Copy List in Python
Related posts:
How to Convert CSV to Tab Delimited File in Python
How to Create Python Function with Optional Arguments
How to Configure Python Flask to be Externally Visible
How to Use Sleep Function in Python
How to Export Pandas Dataframe to Excel
How to Store JSON to File in Python
How to Check if File Exists in Python
How to Export Pandas Dataframe to Multiple Excel Sheets
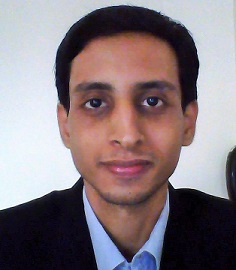
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.