Sometimes you may need to convert webpages into PDF for your application or work. In this article, we will look at how to convert webpage into PDF using Python. We will use wkhtmltopdf & pdfkit libraries for this purpose.
How to Convert Webpage into PDF using Python
Here are the steps to convert webpage into PDF using python.
1. Install pdfkit
Open terminal and run the following command to install pdfkit
$ sudo pip install pdfkit
Also read : How to Create JSON Response Using Django & Python
2. Install wkhtmltopdf
Run the following command to install wkhtmltopdf.
$ sudo apt-get install wkhtmltopdf
Also read : How to Convert CSV to JSON in NodeJS
3. Convert Webpage into PDF
Let us look at different use cases to convert html to pdf. pdfkit provides various functions to convert your content into pdf files. We will look at them one by one.
Convert File into PDF in Python
Here is the command to convert a downloaded web page html document to pdf.
import pdfkit pdfkit.from_file('/home/ubuntu/test.html','output.pdf')
The from_file function in pdfkit library allows you to convert a file into PDF in python. You need to provide the full path to html file and filename of your pdf. If you only provide filename as first argument, it will look for the document in your present working directory.
Also read : How to Set Upstream Branch in Git
Convert URL into PDF in Python
Here is the command to convert a URL into pdf using from_url function.
import
pdfkit
pdfkit.from_url('https://www.google.com','google.pdf')
The from_url function in pdfkit library allows you to convert URL to PDF in python. You need to provide URL as first argument and pdf file’s name as second argument.
Also read : How to Write to File in Bash
Convert String into PDF in Python
Here is the command to convert string into PDF using from_string function.
import
pdfkit
pdfkit.from_string('Hello World','hello.pdf')
The from_string function allows you to convert string into PDF in Python. It takes the string as first argument, and PDF file’s name as second argument.
Also read : How to Disable HTTP Strict Transport Security Policy
Convert multiple files & strings into PDF
You can also use the above commands to convert multiple files, URLs & strings into PDF file. Just use a list of filenames, URLs, and strings as the first argument. Here are the examples
pdfkit.from_file(['/home/ubuntu/test.html','/home/ubuntu/test2.html','/home/ubuntu/test2.html'],'output.pdf')pdfkit.from_url(['https://www.google.com','https://www.facebook.com'],'two-sites.pdf')
pdfkit.from_string(['Hello',' ','World'],'hello.pdf')
In this article, we have learnt different ways to convert our URL, web page and strings into PDF in Python.
Also read : How to Merge Two Dictionaries in Python
Related posts:
How to Reverse/Invert Dictionary Mapping in Python
How to Sort List of Tuples by Second Element in Python
How to Redirect Stdout & Stderr to File in Python
How to Check if Key Exists in Python Dictionary
How to Create Nested Directory in Python
How to Permanently Add Directory to Python Path
How to Import from Parent Folder in Python
Call Python Function by String Name
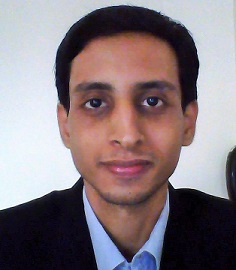
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.