JSON is a popular data type used to exchange data between browser and server. It also supports data exchange across different platforms. In this article, we will look at how to create JSON response using Django, a popular web framework for Python. You can use these steps to make your python backend return JSON data to client browser in websites & web applications.
How to Create JSON Response Using Django & Python
Here are the steps to create JSON response in Django.
Django >= 1.7
Django versions since 1.7 have an in-built function JsonResponse specifically created to help you return JSON response. Here is an example.
from django.http import JsonResponse
def test_json(request):
json_ata = {
'id': 1,
'name': 'John Doe',
'roles' : ['User']
}
return JsonResponse(json_data)
Let us look the above code in details. The first line imports the JsonResponse function from django.http package. Then we define a JSON object json_data in test_json function. Finally, we return this object using JsonResponse function.
Also read : How to Run Shell Script in Linux
Django < 1.7
If you run Django older than 1.7 then you need to use HttpResponse function to return JSON response. Here is the above example using HttpResponse function.
import json
from django.http import HttpResponse
def test_json(request):
json_data = {
'id': 1,
'name': 'John Doe',
'roles' : ['User']
}
return HttpResponse(json.dumps(json_data), content_type="application/json")
Let us look at the above code in detail. First we import json package. Then we define JSON object json_data in function test_json. Finally, we return JSON object using HttpResponse function, which takes two arguments – data to be returned, and its MIME type (content type). Since we are returning json data, the content type needs to be “application/json” always.
Also read : How to Upgrade Django Version
If you run python < 2.7, then you will need to import simplejson package instead of importing json package.
import simplejson
Similarly, instead of using json.dumps, you will need to use simplejson.dumps.
return HttpResponse(simplejson.dumps(json_data), content_type="application/json")
Please note, whether you use JsonResponse or HttpResponse, you will get the same result. This is useful especially when you are upgrading your Django version and need to update your code.
In this article, we have learnt how to return JSON response using Django in Python.
Also read : How to Use NGINX as Reverse Proxy for Django
Related posts:
How to Import Python Modules by String Name
How to Create Superuser in Django
How to Terminate Python Subprocess
Django Get Unique Values From Queryset
How to Enable CORS in Django Project
How to Loop Through List in Django Template
How to Run Python Script in Django Project
How to Delete Objects in Django
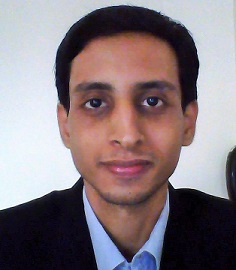
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.