Very often you may need to read GET parameters passed in user requests, while using Django. In this article, we will look at how to capture URL parameters in Django request.
How to Capture URL Parameters in Django Request
There are two ways to pass URL parameters in Django request, and consequently two ways to read them in GET request. We will look at each of them in detail.
1. As Query String
When URL parameters are passed as query string, then you can read them using request.GET function. For example, let us say your site’s URL is like http://example.com/data/?id=15, URL parameter id=15 is passed as a query string.
In this case, you can read the query parameter as request.GET.get(‘id’,”). You can replace id with your query parameter. We use ” as default value if id parameter is not found. Here is an example of request handler for the above URL in views.py.
def test(request): id = request.GET.get('id','') return HttpResponse('id:'+id)
2. As URL Argument
When URL parameters are passed as arguments, then you can directly read them as argument variables in your Django view. For example, if your URL is like http://example.com/data/15, URL parameter 15 is being as argument.
In this case, your URL configuration urls.py can have the following regex based URL definition, as shown below.
url(r'^data/(?P<id>\d+/$', views.test,),
In the above URL configuration we have defined a URL argument id which assumes a numeric value, specified by regex \d+.
In such cases, Django will automatically capture URL parameter id’s value using regular expressions and pass it to function test as named arguments.
In this case, you can modify your test Django view as shown below.
def test(request, id): return HttpResponse('id:'+id)
Please note above, we have mentioned id URL parameter as a function argument. It must be same as the one we have mentioned in our URL configuration within ?P< … >. Otherwise Django won’t know to which function argument, it needs to assign the URL parameter.
We have seen two ways to read URL parameters in Django. Please note that the above methods work only for GET requests and not POST ones.
Also read:
How to Temporarily Disable Foreign Key Constraint in MySQL
How to Lookup Dictionary Value with Key in Django
How to Loop Through List in Django Template
How to Run Python Script in Django
How to Disable CSRF Validation in Django
Related posts:
How to Remove URL Parameters using .htaccess
How to Loop Through List in Django Template
How to Get Classname of Instance in Python
How to Get URL Parameters Using jQuery or JavaScript
How To Upgrade Django Version
How to Disable CSRF validation in Django View
How to Find Django Install Location
How to Create Cartesian Product of Two Lists in Python
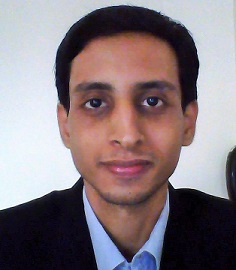
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.