Python provides various modules & functions to help you work with files. Sometimes you may need to get file size in Python. It is useful to get file size for various purposes such as checking attachment size before sending emails, and so on. In this article, we will learn how to get file size in Python.
How to Get File Size in Python
Here are the different ways to get file size in Python.
1. Using os.path module
In this example, we use getsize function of os.path module to get file size in Python.
import os file_path='d:/image.jpg' file_size = os.path.getsize(file_path) print("File Size is :", file_size, "bytes")
In the above code, we first import os module and call os.path.getsize() function to get file size in bytes. The above function returns file size for all types of files such as .txt, .doc, .jpg, .png, etc.
2. Using os.stat
In this case, we use os.stat function to get vital statistics such as size, creation date, modification date, about a given file.
import os file_path='d:/file.jpg' file_size = os.stat(file_path) print("Size of file :", file_size.st_size, "bytes")
In this case, os.stat returns an object with different variables containing different information about file. Since we require file size, we will output st_size variable’s value.
3. Using pathlib module
You can also use pathlib module to get file size. It returns an object that contains information such as st_mode, st_dev and other stats of file. We will use st_size property to find the file size.
from pathlib import Path # getting file size file=Path(r'd:/file.jpg').stat().st_size # display the size of the file print("Size of file is :", file, "bytes")
We use path().stat() to open file for reading, and use its st_size variable to get file size.
In this article, we have learnt different ways to find file size in python. Each of the above method returns file size in bytes. If you want to get file size in kB or Mb, just divide the file size by 1024 or 1024*1024 respectively.
import os file_path='d:/image.jpg' #file size in kB file_size = os.path.getsize(file_path) print("File Size is :", file_size/1024, "bytes")
Typically, file check mechanisms are part of bigger functions or modules where you need to take action if file size is more than a given threshold. So you can modify the above code as per your requirement and embed it in your application or script, as per your requirement.
Also read:
How to Block URL Parameters in NGINX
How to View Active Connections Per User in MySQL
Related posts:
How to Use Sleep Function in Python
How to Merge Two Dictionaries in Python
How to Run Python Script in Django Project
How to Move File in Python
How to Find Local IP Address in Python
How to Find Last Occurrence of Character in String in Python
How to Merge PDF Files Using Python
How to Get Difference of Two Dictionaries in Python
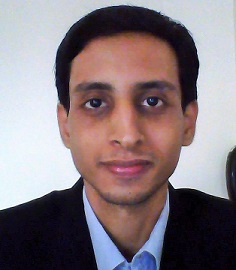
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.