There are plenty of websites and third-party services to determine the IP address of a system. There are also many system tools to determine local IP addresses of system. But sometimes you may need to find local IP address from within your python application or website, using one of the python libraries. This is required if you need to enable/disable features based on IP address and make it accessible only for certain IPs. In this article, we will learn how to find local IP address in Python.
How to Find Local IP Address in Python
Python provides a native library called socket that supports many useful features including IP address determination.
Here is a simple code snippet to find local IP address.
import socket s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) s.connect(("8.8.8.8", 80)) print(s.getsockname()[0]) s.close()
In the above code, we import socket library, and call socket() function to create a socket. Then we connect to Google DNS with IP address 8.8.8.8 and determine the IP address used for the connection to determine the public IP address of local machine.
If the above code, you can also use the following code to find local IP address.
import socket socket.gethostbyname(socket.gethostname())
The above method may not work if the hostname of your system is 127.0.0.1. In such cases it will return 127.0.0.1 as local IP address.
In this article, we have learnt how to get local IP address using python. You can use it to determine IP address of system from within python script, application or website.
Also read:
How to Fix ValueError: Invalid Literal for Int With Base 10
How to Drop Pandas Rows with NaN Values
How to Run Python Script from PHP
How to Convert All Strings in List to Integers
How to Uninstall Python 2.7 on Mac OS
Related posts:
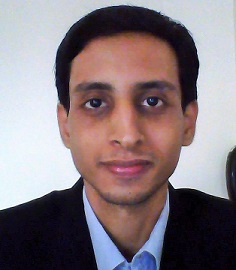
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.