Sometimes while working with Python, you may get the error ‘ValueError: invalid literal for int() with base 10’. This error generally occurs when you try to convert a string representation of floating point number to an integer, or a string representation of anything except an integer (like empty string) into an integer. In this article, we will learn how to fix this problem.
How to Fix ValueError: invalid literal for int() with base 10
Let us say you try the following command.
int('5.0')
You will get the following error.
Traceback (most recent call last): File "<pyshell#0>", line 1, in <module> int('5.0') ValueError: invalid literal for int() with base 10: '5.0'
So a simple way to solve this problem is to call float() function on the floating point number before calling int() function on it.
int(float('5.0')) 5
If your string is possibly empty, then it is better to test for an empty string before calling int() function.
a='' if a != '': int(a) else: print 'empty string'
In this article, we have learnt a couple of simple ways to fix ValueError: invalid literal for int() with base 10 in Python.
Also read:
How to Drop Rows in Pandas with NaN
How to Run Python Script in PHP
How to Convert All Strings in List to Integer
How to Uninstall Python 2.7 on Mac OS
How to Parse URL into Hostname & Pathname
Related posts:
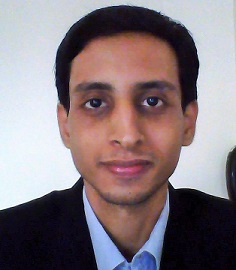
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.