JavaScript objects allow you to store different types of data in one place as key-property pairs. You can easily access property values using their keys. But if you try to access property from empty object, you will get an error, and it might stop code execution. So it is advisable to check if an object is empty before you access its properties. In this article, we will learn how to check if object is empty in JavaScript.
How to Check if Object is Empty in JavaScript
You can check for empty JS object using plain JavaScript or using the JS library that you prefer. They all support this feature. We will look at how to do this in different ways.
1. Using JavaScript
Let us say you have an object obj then here is the JavaScript command to check if it is empty or not.
obj && Object.keys(obj).length === 0 && Object.getPrototypeOf(obj) === Object.prototype
The above command will return an array of key values which will be empty if the object is empty.
2. Using Jquery
If you are using jquery on your website, then it provides a really easy way to check for empty objects.
jQuery.isEmptyObject(obj);
The above command will return true if the object is empty, else false.
3. Using Lodash
If you use lodash library on your website, use the following command to determine if an object is empty or not.
_.isEmpty(obj);
The above command will return true for empty objects, false otherwise.
4. Using AngularJS
You can also use the popular AngularJS library to check if JS object is empty or not.
angular.equals(obj, {});
In the above command, AngularJS basically compares our given object obj with an empty dictionary and returns true if they are both equal, that is, our object is empty, else false.
5. Using ExtJS
If you use ExtJS on your website/application, you can use the following command to check if a JS object is empty.
Ext.Object.isEmpty(obj);
The above command returns true if the object is empty, else it returns false.
6. Using Underscore
If you use Underscore library, you can use the following command to check if JS object is empty or not.
_.isEmpty(obj);
The above command returns True if the object is empty, false otherwise.
7. Hoek
If you are using Hoek library, you can use the following command to determine if an object is empty.
Hoek.deepEqual(obj, {});
Like AngularJS, it also compares our object with empty object and returns true if they are both equal, that is, the object is empty, else it returns false.
8. Using Ramda
In Ramda library, you can use the following command to check for empty objects.
R.isEmpty(obj);
The above command returns true if the object is empty, else it returns false.
In this article, we have learnt several ways to check if JS object is empty or not. You can use any of them as per your requirement. As you can see, it is easier to check for empty objects if you use a built-in function available in your JS library, instead of using plain JavaScript.
This is very useful in avoiding errors due to empty objects. For example, if you are getting a JSON response from server and converting it into JS object for processing, it is better to check if the object is empty to avoid errors and termination of execution.
Also read:
How to Return Response from Asynchronous Call in JavaScript
How to Remove Key from JavaScript Object
How to Deep Clone Objects in JavaScript
How to Include JavaScript File in Another JavaScript File
How to Add Event to Dynamic Elements in JavaScript
Related posts:
How to Get URL Parameters Using jQuery or JavaScript
How to Detect Invalid Date in JavaScript
How to Deep Clone Objects in JavaScript
How to Convert Array to Object in JavaScript
How to Call JS Function in IFrame from Parent Page
How to Get Image Size & Width Using JavaScript
How to Generate Random Color in JavaScript
How to Check if JavaScript Object Property is Undefined
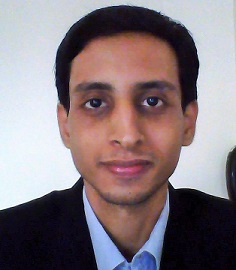
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.