JavaScript is a popular front end language that allows web developers and programmers to easily build websites. Often web developers may need to make the first letter upper case in a string or sentence. In this article, we will learn how to capitalize first letter in JavaScript.
How to Capitalize First Letter in JavaScript
Here are the steps to capitalize first letter in JavaScript. Let us say you have the following string in JavaScript.
str = "jim"
We will use toUpperCase() function to convert the first character to upper case. This function converts all characters in a string to upper case, so we will call this function only on the first character. We will use charAt(0) function to get the first character that is the character at position 0.
str.charAt(0).toUpperCase() //J
To the above result, we will append rest of the unmodified string. For this purpose, we will use slice() function. slice() function allows you to extract a substring from a given string by mentioning the starting character, and optionally specifying the last character
str.slice(1) //im
We will concatenate the two above results to get our desired string.
str.charAt(0).toUpperCase() + str.slice(1);
Putting together everything, we will create a simple function to help us convert any input string’s first character as capital letter.
function capitalizeFirstLetter(string) { return string.charAt(0).toUpperCase() + string.slice(1); }
Here is how to call the function.
console.log(capitalizeFirstLetter('jim')); // Jim
If you want, you can also add this function to String prototype so that it is easy to call.
String.prototype.capitalize = function() { return this.charAt(0).toUpperCase() + this.slice(1); }
Once you have defined the prototype function, you can easily call it directly from any string as shown below.
str = 'jim'; console.log(str.capitalize()); //Jim
In this article, we have learnt how to capitalize the first letter of string in JavaScript. You can include it as a part of a bigger module or run it as a standalone function.
Also read:
Python Run Shell Command & Get Output
How to Generate All Permutation of Python List
How to Sort List of Dictionaries by Value in Python
How to Count Occurrence of List Item in Python
How to Restore Default Repositories in Ubuntu
Related posts:
How to Add 1 Day to Current Date
How to Count Substring Occurrence in String in JS
How to Break ForEach Loop in JavaScript
How to Pass Parameter to SetTimeout Callback
How to Get Element's Outer HTML using jQuery
How to Close Current Tab in Browser Window
How to Count Frequency of Array Items in JavaScript
How to Add Days to Date in JavaScript
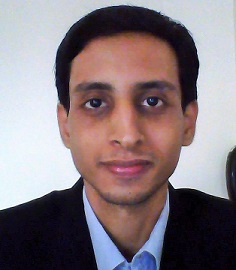
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.