Often you may need to read file line by line in Linux shell script. In this article, we will look at different use cases for reading file in shell script.
Suppose you have a file /home/ubuntu/data.txt with the following information
Today is a beautiful day
How to Read File Line by Line in Linux Shell Script
Here are the different ways to read the above file line by line.
1. Read File from Command Line
If you don’t want to write a shell script or even use cat command to view your file contents, you can use a while loop in terminal prompt to simply go through the file line by line and display its contents.
$ while read line; do echo $line; done < /home/ubuntu/data.txt
In the above code, the while loop goes through the file. Each line is temporarily stored in $line variable. We use echo command to display the line. Please note, if you don’t use the full path below, it will look for the file in your present working directory.
$ while read line; do echo $line; done < /home/ubuntu/data.txt Today is a beautiful day
2. Read File using Shell Script
Here is a simple shell script to read the file and display its contents line by line.
$ sudo vi read_file.sh
Once you have created an empty shell script with the above command, add the following lines to it.
#!/bin/bash filename='/home/ubuntu/data.txt' n=1 while read line; do # reading each line echo "Line No. $n : $line" n=$((n+1)) done < $filename
In the above code, we store file path in $filename variable. Then we run a while loop through the file, storing each line in $line variable. We also use $n variable to keep count of line number.
Make the shell script executable
$ sudo chmod +x read_file.sh
Run the shell script using the following command.
$ ./read_file.sh
If you want to pass the filepath via command line argument, replace the second line of your script as
filename=$1
In the above code, $1 stores the first command line argument. Now your shell script will be
#!/bin/bash filename=$1 n=1 while read line; do # reading each line echo "Line No. $n : $line" n=$((n+1)) done < $filename
Now you can run your shell script file as
$ ./read_file.sh /home/ubuntu/data.txt
3. Omit backslash escape character
Please note, if your file contains backslash then the above code will treat it as escape character. If you want to omit backslash escape, then you need to use -r option with read command in your while loop, as shown below.
#!/bin/bash while read -r line; do # Reading each line echo $line done < /home/ubuntu/data.txt
In this article, we have learnt different ways to read file line by line shell script. Reading files is a very common requirement in Linux and can be used in many bigger scripts. If you want to perform any operation on each read line, then you can include those statements between while..done statements.
You can use these commands with any shell. In case you use any of the above shell scripts with other shell, please update the first line to point to the right shell.
Also read:
How to Recover Deleted Files in Linux
How to Use Key-Value Dictionary in Shell Script
How to Block Website in Linux
How to Clear Terminal History in Linux & MacOS
How to Show Asterisk for Password in Linux
Related posts:
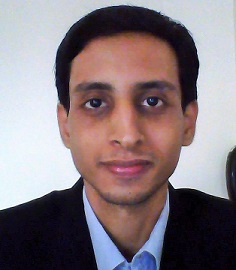
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.