Sometimes you may need to execute certain shell commands or shell scripts from within your python script/application. There are multiple ways to do this. In this article, we will look at the different ways to execute shell command from python.
How to Execute Shell Command from Python
Here are the different ways to execute shell command from python. In python, os and subprocess module provide the functions to run shell commands. We will be looking at the different functions available in these packages for our purpose.
Using OS module
The simplest way to run a shell command (e.g. “ls -a”) is to use os.system() function. Here is an example
import os os.system("ls -a")
In the above code, we first import os module and then use os.system() to run the shell command within quotes.
You can even use it to pipe shell commands as shown below.
import os os.system("ls -a | grep file.txt")
Please note, os.system() does not return any output so you can only run shell commands with this but not use its output for further processing.
If you need to read the result of shell command’s output, use os.popen() function as shown below.
import os s=os.popen("echo hello world") o=s.read() o hello world
In the above code, os.popen() returns a stream to the shell commands to output sent to stdout. You can read it, display it and work with it further if you want. It is very useful for piping the output of one command to python functions.
Using subprocess
subprocess module provides comprehensive functions to work with shell commands. It provides subprocess.call() on Python <=3.4 and subprocess.run() on python 3.5 and above to run shell commands and scripts. Here is an example.
import subprocesssubprocess.call(["ls", "-l"])
#python <=3.4subprocess.run(["ls", "-l"])
#python >= 3.5
It provides more powerful options to create new processes and retrieve results that you can easily use further. For example, it also provides subprocess.popen() function that makes it easy to read shell command outputs. Here is an example.
import subprocess
p = subprocess.Popen(['echo', 'hello world'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = p.communicate()
stdout, stderr
However, subprocess functions have more complicated syntax, than those of os module, since they are more versatile and comprehensive.
If you need to run simple shell commands and scripts, try os module. If you need something more complicated (like retrieving stderr, status code, error handling, etc), then go for subprocess module. Having said that, the recommended way to run shell commands and scripts in python, is to use subprocess module.
Also read:
How to Copy Files from One Directory to Another
Grep: Exclude Files & Directory
Install Fail2ban in CentOS 7
How to Copy File to Multiple Directories in Linux
How to Run Python Script in Django
Related posts:
How to Get Classname of Instance in Python
How to Sort List of Tuples by Second Element in Python
How to Create Nested Directory in Python
How to Extract Numbers from String in Python
How to Setup Uwsgi with NGINX for Python
How to Remove Duplicates from List in Python
How to Create Multiline String in Python
How to Create Nested Directory in Python
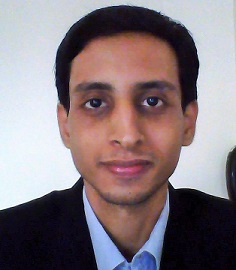
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.