Sometimes you may need to iterate over multiple lists together or simultaneously. There are different ways to do this in python. In this article, we will look at how to iterate multiple lists in parallel in Python.
How to Iterate over Multiple Lists in Parallel in Python
You can use either zip() or itertools.zip_longest() functions to iterate over multiple lists in parallel in Python.
1. Using zip()
zip() function traverses both lists in parallel but stops the moment any of the individual list is exhausted. In other words, zip() stops when the shortest list in the group stops. Here is an example to iterate over 2 lists in parallel using zip() function. It returns a list of tuples, where each tuple contains 1 item from each of the lists being iterated.
>>> num1 = [1, 2, 3] >>> num2 = [4, 5] >>> for (a, b) in zip(num1, num2): print (a, b) (1, 4) (2, 5)
Please note, zip() function runs only till the smallest list runs.
Also is you are using really long lists, then you should use itertools.izip() instead to save memory and for better performance. This is because in python 2.x izip() returns an iterator while zip() returns list.
>>> import itertools >>> num1 = [1, 2, 3] >>> num2 = [4, 5] >>> for (a, b) in itertools.izip(num1, num2): print (a, b) (1, 4) (2, 5)
2. Using itertools.izip_longest()
If you want python to traverse till the longest list is exhausted, then use itertools.izip_longest() function instead.
>>> import itertools >>> num1 = [1, 2, 3] >>> num2 = [4, 5] >>> for (a, b) in itertools.izip_longest(num1, num2): print (a, b) (1, 4) (2, 5) (3, None)
In this article, we have learnt different ways to traverse two lists in parallel. zip() function iterates the lists till any of them gets exhausted. itertools.izip_longest() runs till all lists are exhausted. If you are using python 2.x and your lists are huge then you need to use itertools.izip() that returns an iterator, thereby saving memory.
Please note, in python 3.x, izip() and izip_longest() are not there as both zip() and zip_longest() return iterators. In python 2.x, zip() used to return lists.
Also read:
How to List All Files in Directory in Python
How to Send HTML Email with Attachment in Python
Postfix Mail Server Configuration Step by Step
How to Install Go Access Log Analyzer in Ubuntu
How to Check Python Package Version
Related posts:
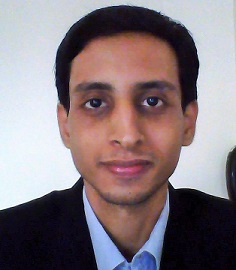
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.