Sometimes you may need to open file dialog box in your python application. There are many python libraries and modules to help you do that. But tkinter offers one of the easiest ways to work with GUI elements. In this article, we will learn how to open file dialog box in python, using tkinter.
How to Open File Dialog Box in Python
Tkinter is generally pre-installed in all Python distributions so there is no need to any setup. You can simply add the following lines to your code, depending on your Python version, to open file dialog box.
Here is the code for python 3.x.
import tkinter as tk from tkinter import filedialog root = tk.Tk() root.withdraw() file_path = filedialog.askopenfilename()
In the above code, we first import tkinter and use its filedialog function. We define root element and then hide it using withdraw function. Finally, we use askopenfilename() function to open file dialog box.
If you have python 2.x, you need to use the following code.
import Tkinter, tkFileDialog root = Tkinter.Tk() root.withdraw() file_path = tkFileDialog.askopenfilename()
It has similar approach like the previous code block. In this case, we use tkFileDialog object (python 2.x) instead of using filedialog object (Python 3.x).
In this short article, we have learnt how to open file dialog box in Python.
Also read:
How to Share Folders Between Linux Servers
How to Disable SELinux in CentOS & RHEL
How to Setup SSH Tunneling in Linux
How to Save Terminal History in Linux
How to Delete All Instances of Character in Python String
Related posts:
How to Combine Multiple CSV Files Using Python
How to Check if Substring is in List of Strings
How to Find Index of Item in List in Python
How to Setup Uwsgi with NGINX for Python
How to Export Pandas Dataframe to Multiple Excel Sheets
How to Raise Exception in Python
How to Split String by Delimiter in Python
How to Import Other Python File
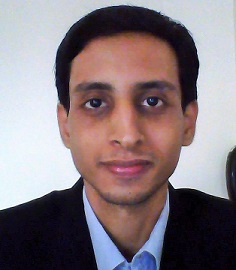
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.