Sometimes you may need to convert a list of strings or integers into a string in Python. There are different ways to convert list to string. In this article, we will look at how to convert list to string in Python.
How to Convert List to String in Python
We will look at four different ways to convert list to string in Python.
1. Using join function
Let us say you have the following list of strings. You can use the join function directly to join them into a string. Join function takes an iterable and joins them into a string.
Here is the syntax of join function
string.join(iterable)
In the above function, the string will be used a separator while joining the individual elements of the iterable such as list, tuple, etc.
Here is an example to convert list to string without commas.
>>> a=['apple','banana','cherry'] >>> b=' '.join(a) >>> b 'apple banana cherry'
Here is an example to convert list to string with commas. Just change the string used in join function to be ‘,’.
>>> a=['apple','banana','cherry'] >>> b=','.join(a) >>> b 'apple banana cherry'
You can also use join function to join elements of a tuple into a string. Please note, join function works only on string items. It will not work if you have a list of integers. In that case, you need to use the next method.
Also read : How to Install HAProxy in Ubuntu
2. Using List Comprehension
In this case, you can join a list of integers, or even lists that contain both integers and strings. Here is an example
>>> a=[1,2,3] >>> b=' '.join([str(elem) for elem in a]) #using list comprehension >>> b '1 2 3'
In the above list comprehension on line 2, we loop through the list and convert each element into a string before joining them.
Here is an example to convert a list containing both integers and strings into a string.
>>> a=['I','want',10,'dollars'] >>> b=' '.join([str(elem) for elem in a]) >>> b 'I want 10 dollars'
Also read : How to Run Scripts on Startup in Ubuntu
3. Using map function
Similarly, you can use a map function to convert a list to string.
>>> a=['I','want',10,'dollars'] >>> b=' '.join(map(str,a)) >>> b 'I want 10 dollars'
In the above code, we map str function to each element of list a, and then call join function on it.
Also read : How to Import CSV in Python
4. Using for loop
Lastly, you can do the above conversion using good old fashion for loop, as shown below.
>>> a=['I','want',10,'dollars'] >>> b='' >>> for i in a: b+=str(i)+' ' >>> b 'I want 10 dollars '
Also read : How to Install Tomcat in Ubuntu
That’s it. As you can see it is very easy and quite fun to convert list into strings. In this article, we have described four different ways to convert list to string. Hope you find them useful.
Related posts:
What Does __file__ Mean in Python
How to Execute Stored Procedure in Python
Python Script to Check URL Status
How to Convert PDF to Text in Python
Python Script to Run SQL Query
How to Remove HTML Tags from CSV File in Python
How to Flatten List of Dictionaries in Python
How to Drop One or More Columns in Python Pandas
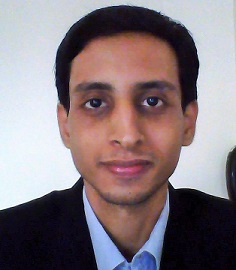
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.