Python is a powerful programming language that allows you to do a lot of things. You can even use it to backup files & folders on your system. In this article, we will learn how to automate backup in Python. We will use shutil, os & sys libraries for this purpose. shutil is the main library used to copy files & dir_util is the main module to copy folders from one location to another, while os libraries are used to obtain file & folder paths.
How to Automate Backup in Python
Here are the steps to automate backup in python. Create an empty python script file for this purpose.
$ sudo vi backup.py
1. Import Modules
First, we import the required modules. We will use shutil to copy individual files and copy_tree to copy folders.
import shutil from datetime import date import os from distutils.dir_util import copy_tree
2. Get Latest Date
Typically, we add a datetime stamp to our backup file. For this purpose, we will calculate the present date value. We will use datetime library for this purpose. You can change the date format as per your requirement.
today = date.today() date_format = today.strftime("%d_%b_%Y_")
3. Enter Source & Destination Locations
We will build a script that allows you to backup single file in a folder, as well well all files in folder. So we use 4 variables for source folder, source file, destination folder and destination file.
src_dir = <enter full source folder path here> src_file = <enter source filename here> dest_dir = <enter full destination folder path here> dest_file = <enter destination filename here>
4. Copy Files & Folders
Add the following code to backup files & folders from source to destination. We will first check if the source filename is empty. In that case, we will copy all files in source folder to destination folder. If source file is specified, then we will copy it to destination file.
try: if dest_folder=="": print "enter destination folder location" return #copy entire folder if src_file=="": copytree(src_dir, dst_dir) return #copy single file if dest_file=="": dest_file=src_file shutil.copy2(os.path.join(src_dir,src_file), os.path.join(dst_dir,dest_file+date_format)) return except FileNotFoundError: print("File does not exists!,\ please give the complete path")
In the above code, we use a try…catch block to make sure that is a file or folder is not found, then we catch those errors. Inside our try block, we first check if the destination folder is provided or left blank. If it is blank, we ask user to enter destination folder. Next, we check if source filename is provided. If not, then we copy the entire source folder to destination folder. Finally, if destination file is left blank, we give it the same name as source file and append datetime stamp to destination filename. We use os.path.join function to concatenate folder location and filename, in case we need to transfer files. You may also use simple string concatenation for this purpose.
You may also add the above try…catch block in a function if you want.
5. Run the Python Script
Save and close the file. Run the script with the following command.
$ sudo python backup.py
In this article, we have learnt how to backup files & folders in Python. You can modify it as per your requirements, or even embed it as a part of a bigger module in your applications.
Also read:
How to Check Long Running Processes in Linux
How to Backup & Restore hard Disk in Linux
How to Lock & Unlock Users in Linux
How to Change FTP Port in Linux
How to Check CVE Vulnerability in Linux
Related posts:
How to Create Cartesian Product of Two Lists in Python
How to Drop One or More Columns in Python Pandas
How to Check if Variable is Number in Python
How to Redirect Stdout & Stderr to File in Python
How to Create Python Function with Optional Arguments
How to Call C Function in Python
How to Compare Dictionary in Python
How to Check if Directory Exists in Python
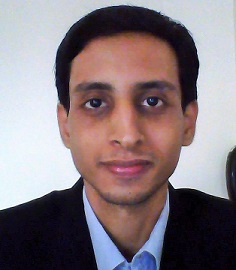
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.