Lists are one of the most commonly used data structures in Python. They are very powerful and offer many useful features. Often python developers work with multiple lists and may need to get difference between two lists in Python. It can be tedious to loop through both the lists to get their differences. There are simpler ways to do this. In this article, we will learn how to get difference between two lists in Python.
How to Get Difference Between Two Lists in Python
Let us say you have the following two python lists.
temp1 = [1, 2, 3, 4] temp2 = [1, 2]
Let us say you want to get difference between the two above lists as [3, 4]. You can easily do this by converting each list into a set using set() function and then subtracting second set from the first. The result is also a set, so we need to use list() function to convert the output back to a list
list(set(temp1) - set(temp2)) [3, 4]
In the above code, we need to convert lists into sets, because subtraction and difference operations are supported by only sets and not lists. This operation is similar to getting a difference between two sets in mathematics.
Please note, the above difference is asymmetric, that is, if you subtract the first list from second it will give a different result.
list(set(temp2) - set(temp1)) []
The above operation gives null list because there is no element in temp2 which is not present in temp1.
Similarly, let us say you have the following two lists
t1 = [1, 2] t2 = [2, 3]
Look at the result of both the differences, one where we subtract t2 from t1 and other where we subtract t1 from t2.
list(set([1, 2]) - set([2, 3])) [1] list(set([2, 3]) - set([1, 2])) [3]
In the above case, if you are looking for the result as [1, 3] then you need to do a symmetric difference as shown below. In this case, you need to use symmetric_difference() function.
list(set([1, 2]).symmetric_difference(set([2, 3]))) [1, 3]
In the above case, it will return a list of elements not present in both the lists, that is those elements present in t1 but not t2 and those present in t2 but not t1.
In this article, we have learnt several ways to get difference of two lists in Python. The key is to convert lists to sets and then get their difference. Since the result of difference of two sets is also a set, we use list() function to convert the result set back to list.
Also read:
How to Import Other Python File
How to Remove Punctuation from String in Python
How to Do Case Insensitive String Comparison in Python
How to Remove Duplicates from Array of Objects JavaScript
How to Listen to Variable Changes in JavaScript
Related posts:
How to Get Key from Value in Python Dictionary
How to Print Without Newline or Space in Python
How to Schedule Task in Python
What Is __name__ In Python
How to Get Difference of Two Dictionaries in Python
How to Execute Shell Command from Python
How to Remove All Occurrences of Value from List in Python
How to Concatenate Multiple Lists in Python
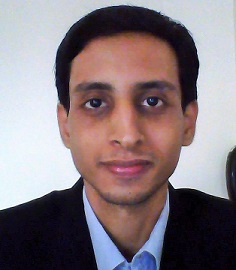
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.