Print is a commonly used python function to print data. But the print function in python adds a newline or blank space without being specified by user. Sometimes you may need to print lines in python without newline or space. In this article, we will learn how to print without newline or space in python.
How to Print Without Newline or Space in Python
By default, when you print data one after the other in python, it will insert a blank space in between.
>>> print('.', '.', '.', '.') . . . .
When you print data line by line, it inserts newline character.
>>> for i in range(4): print('.') . . . .
Addition of newline or space seems unnecessary to many. For this purpose, you can use sep and end parameters.
To avoid adding blank space between print items, mention sep=” in print function as last argument. It sets the separator to empty character, instead of using blank space.
print('a', 'b', 'c', sep='') abc
On the other hand, if you don’t want to add newline after every line, then specify end=” argument in print function.
>>> for i in range(4): print('.',end='')
When you use end=” print will set of end of line character as empty string and not newline character.
You can pass any string or character as sep or end parameters. You can even use both at the same time.
If you are facing problems with buffering, you can use flush=True parameter in print function to make it work.
print('.', end='', flush=True)
The above solutions work with python 3+. If you are using python 2.6 or 2.7, then you need to import print function using __future__ module.
from __future__ import print_function
But please note, the flush feature works on python 3 only and not earlier versions.
In this article, we have learnt how to print in python without newline or whitespace characters in between.
Also read:
How to Convert String Representation to List in Python
How to Set Current Working Directory in Shell Script
How to Change Shutdown Message in Linux
How to Mkdir Only If Directory Does Not Exist
How to Create Nested Directory in Linux With One Command
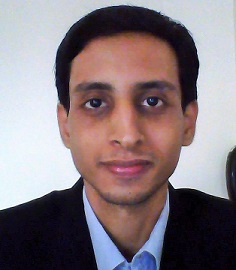
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.