By default, when users close tab/window while they are on your website, their browser closes it without showing any confirmation dialog box. Sometimes you may need to display an alert or confirmation dialog box when the user tries to close current browser tab/window while being on your website. Otherwise, people may end up leaving your site, even if they accidentally close the tab/window. In this article, we will learn how to do this.
How to Close Current Tab in Browser Window
The JavaScript code to close current browser tab is
close() OR window.close()
In both the above commands, the current tab is implied.
We can use this to create a function that displays a confirmation box before closing the window.
function close_window() { if (confirm("Are you sure?")) { close(); } }
Now we can call this function when a button is clicked.
<a href="javascript:close_window();return false;">close</a> OR <a href="#" onclick="close_window();return false;">close</a>
Both the above commands call close_window() function when the URL is clicked. We return false so that its default behavior is prevented, and close_window() is called. Otherwise, browser will try to take you to the URL’s destination.
window.confirm() function displays a simple Ok/Cancel dialog box with the input message as the title. If you want to display something else, you can probably use a modal with customization.
Please note, some browsers like Firefox do not allow you to close other browser windows, using any method. Also recent browsers do not allow you to close tab/window using JavaScript, unless they were opened using window.open() JaavaScript function. So it is advisable that if you want to close a tab/window using JavaScript, first open it using window.open(). You can call this function when a link/button is clicked, instead of allowing browser to open the page directly for you. Once your page is opened this way, you will be able to close it using window.close() function.
Here is a complete example. Let us say your page has the following two buttons – one to open a page using window.open() and the other to close it using window.close().
<button onclick="openWin()">Click to open</button> <button onclick="closeWin()">Click here to close the window</button>
Here is the JavaScript code to open page on clicking 1st button and close the same page on clicking the 2nd button.
<script> var test; function openWin() { test = window.open("https://www.fedingo.com", "_blank", "width=786, height=786"); } function closeWin() { test.close(); } </script>
In the above code, closeWin() function uses the same variable that was used to store the information of window opened using window.open() function in openWin() function.
In this article, we have learnt how to close current tab/window in browser using JavaScript. It is useful if you want to prevent users from closing tab/page, without a confirmation, while they are on your site.
Also read:
How to Get ID of Element That Fired Event in jQuery
How to Get Property Values of JS Objects
How to Scroll to Bottom of Div
How to Remove Empty Element from JS Array
How to Check if Variable is Array in JS
Related posts:
How to Get Client IP Address Using JavaScript
How to Get Subset of JS Object Properties
How to Find Sum of Array of Numbers in JavaScript
How to Render HTML in TextArea
How to Add 30 Minutes to JS Date Object
How to Convert Array to Object in JavaScript
How to Detect When User Leaves Page
How to Compare Two Dates Using JavaScript
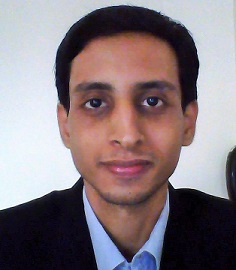
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.