By default, NodeJS has a timeout value of 120 seconds. Sometimes you may need to increase request timeout in NodeJS to process long running requests. In this article, we will look at how to increase request timeout in NodeJS.
How to Increase Request Timeout in NodeJS
Here are the steps to increase request timeout in NodeJS.
If you have not installed NodeJS on your Linux system, use the following command to install it.
#Ubuntu/Debian $ sudo apt install nodejs #Redhat/CentOS/Fedora $ sudo yum install nodejs
Also read : How to Enable HTTP Strict Transport Security in NodeJS
1. Create Server
Open terminal and run the following command to create a file server.js for server-related code.
$ sudo vi server.js
We will use express module to create a server. Add the following to create a NodeJS server.
var express = require('express'); var app = express(); app.listen(80);
The above code will import express module and create a server that runs on port 80.
Also read : How to Run NodeJS on Port 80
2. Increase Request Timeout
We will use the setTimeout method for response objects to set the timeout value for our server. For example, if you want to set request timeout to 200 seconds, then add the following line to server.js above.
res.setTimeout(200);
Please note, we use res.setTimeout here because our response object is defined as res below. If your response object is called resp then you need to add resp.setTimeout(200).
For example, if you want to increase timeout value for all requests to your server, add the above line to app.use function, as shown below.
app.use(function(req, res, next) { res.setTimeout(200); next(); });
If you want to increase request timeout for only a specific URL or request, then add res.setTimeout line to app.get or app.post function for that URL, depending on whether its a GET or POST function, as shown below. Here is an example to increase timeout for /product
app.get('/product', function(req, res){ res.setTimeout(200) ... });
Please note, if you want to completely disable request timeout in NodeJS, then use 0 in setTimeout function as shown.
res.setTimeout(0);
Save and close the file.
Also read : Apache HTTP Server vs Apache Tomcat
3. Run NodeJS Server
Run the following command to run NodeJS server.
$ sudo node server.js
Now the request timeout value on your website will have changed.
Also read : How to Enable CORS in NodeJS
Related posts:
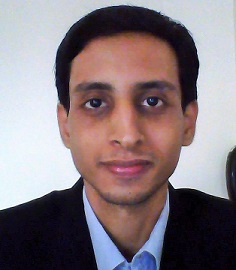
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.