Cross Origin Resource Sharing (CORS) allows your web server to accept requests from other domains and websites. It is useful for setting up APIs and plugins on your website/application. However, it is disabled by default in every web server. In this article, we will look at how to enable CORS in NodeJS.
How to Enable CORS in NodeJS
Here are the steps to enable CORS in NodeJS. Basically, you need to a response header “Access-Control-Allow-Origin” to enable cross domain requests.
If you have not installed NodeJS run the following command to install it on your system.
#Ubuntu/Debian $ sudo apt install nodejs #Redhat/CentOS/Fedora $ sudo yum install nodejs
1. Create Server
Open terminal and run the following command to create a file server.js for server-related code.
$ sudo vi server.js
Also read : Apache HTTP Server vs Apache Tomcat
2. Enable CORS in NodeJS
Add the following lines of code to server.js. We will be using express module to create our server. So we will import it first.
var express = require('express'); var app = express(); app.listen(80);
Next, if you want to enable CORS for all requests on your website, then add the following lines to server.js, depending on your requirement.
Enable CORS for all requests from all domains
If you want to enable CORS for all requests, from all domains, add the following code snippet to server.js.
app.use(function(req, res, next) { res.header("Access-Control-Allow-Origin", "*"); next(); });
Enable CORS for all requests from specific domain
Add the following lines to enable CORS from specific domain such as example.com. Replace * above with allowed domain
app.use(function(req, res, next) { res.header("Access-Control-Allow-Origin", "example.com"); next(); });
If you want to allow CORS from multiple domains, add separate headers for each domain as shown below.
app.use(function(req, res, next) { res.header("Access-Control-Allow-Origin", "example1.com"); res.header("Access-Control-Allow-Origin", "example2.com"); next(); });
If you enable CORS for all requests as shown above, then you don’t need to enable it again for specific URLs as shown below.
Also read : How to Serve Static Files in NodeJS using NGINX
Enable CORS for specific requests or URL route
If you want to enable CORS for only specific request or URL route such as /product, and not all requests, then add the following lines. In this case, you need to add response header only for that URL’s processing as shown below.
app.get('/product', function(req, res){ res.header("Access-Control-Allow-Origin", "*"); ... });
Again, if you want to allow CORS from specific domain, replace * with that domain name above.
app.get('/product', function(req, res){ res.header("Access-Control-Allow-Origin", "example.com"); ... });
Similarly, if you want to allow CORS from multiple domains to a specific URL, then add a separate response header for each domain.
app.get('/product', function(req, res){ res.header("Access-Control-Allow-Origin", "example1.com"); res.header("Access-Control-Allow-Origin", "example2.com"); ... });
Save and close the file.
Also read : How to Run NodeJS on Port 80
3. Run NodeJS Server
Run the following command to start server on port 80.
$ sudo node server.js
That’s it. You can use an online tool like Test-CORS to check if your website is allowing cross domain requests. In this article, we have learnt how to enable CORS in NodeJS. We have also looked at the different use cases for enabling cross domain requests. It is important to determine whether you want to enable CORS for all requests or only specific requests. If you want to enable CORS for all requests, add “Access-Control-Allow-Origin” header in app.use function. If you want to enable cross domain requests, add the header in app.get or app.post functions for those URLs only.
Also read : How to Enable HTTP Strict Transport Security Policy in NodeJS
Related posts:
How to Uninstall NodeJS, NPM Completely in Ubuntu
How to Write to File in NodeJS
How to Read POST Data in NodeJS
How to Run NodeJS on Port 80
How to Convert Callback to Promise in NodeJS
How to Fix EADDRINUSE error in NodeJS
How to Read Command Line Arguments in NodeJS
How to Enable HTTP Strict Transport Policy in NodeJS
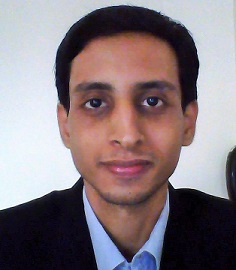
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.