Sometimes when you try to run your NodeJS server you may get an error message “EADDRINUSE” and your server may not start. This error occurs because there is already another process running on the port that your NodeJS server wants to run on. In this article, we will look at how to fix EADDRINUSE error in NodeJS.
How to Fix EADDRINUSE error in NodeJS
You basically need to identify the process running on the port your NodeJS server wants to run and kill it. Sometimes, it may also happen that another instance of your NodeJS server is already running on the same port.
Let us say you have the following NodeJS server app.js that runs on port 80.
var express=require('express'); const app = express(); app.get('/',(req,res) => { res.send('Hello World'); }) const PORT = 80; app.listen(PORT,() => { console.log(`Running on PORT ${PORT}`); })
So you need to find the process on this port number using either of the following commands. Replace 80 below with the port number of your NodeJS server, in case it is different.
$udo netstat -nlp | grep :80
tcp 0 0 0.0.0.0:80 0.0.0.0:* LISTEN 1449/nginx: master OR $sudo lsof -n -i :80 | grep LISTEN
nginx 1449 root 10u IPv4 24508 0t0 TCP *:http (LISTEN)
The above commands should give you the PID (e.g. 1449) of process running on port 80. We have shown it in bold for your reference.
Once you have the PID of process running on your desired port, use kill -9 command to kill the process.
$ sudo kill -9 1449
Now try running your NodeJS server.
$ sudo node app.js
It should run properly this time.
Also read :
How to Use ES6 Import in NodeJS
How to Prevent Direct Access to PHP File
How to Download File in NodeJS Server
How to Fix NGINX Upstream Timed Out Error
How to Fix Permission Denied Error in Django
Related posts:
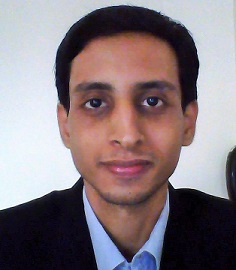
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.