Sometimes you may need to downoad file from NodeJS server when you access a URL on your website. In this article, we will look at how to setup a request handler in NodeJS server so that when your users access a URL, it will immediately offer a file download.
How to Download File from NodeJS Server
Here are the steps to provide a file download from NodeJS server. We will use ExpressJS for this purpose since it has many helper functions to make this task easier.
1. Create NodeJS Server
Create server.js to be run a NodeJS server.
$ sudo vi server.js
Add the following lines to it.
var express = require('express'); var app = express();
The above code will import ExpressJS.
Next add the following lines to parse request body properly, dependin on ExpressJS version on your system.
ExpressJS >=4.16
app.use(express.bodyParser());
ExpressJS < 4.16
const bodyParser = require("body-parser");
app.use(bodyParser);
Next, add the following line to run server on port 80.
app.listen(80);
2. Create Request Handler
Let us say you want your users to be able to download file /uploads/file.pdf when they access /download URL on your web server. In such case, add the following request handler.
app.get('/download', function(request, response){ const file = `${__dirname}/uploads/file.pdf`; response.download(file); // Set disposition and send it. });
Let us look at the above code line by line.
The first line defines the request handler for /download URL.
The 2nd line defines the file path of the file to be offered for download. Please note, we use a NodeJS system variable __dirname which automatically points to the directory where the present file server.js is located. For example, if server.js is located at /home/test then that is the value of ${__dirname}. In our case, server.js and uploads folder are located in same directory. If that is not the case for you, then you may need to update the path according to your requirement. You may also define the file path as absolute file path, without using __dirname variable.
The last line simply sets the content type and sends the file for download.
3. Start NodeJS Server
Open terminal and run the following command to start NodeJS server.
$ sudo node server.js
Open browser and visit http://localhost/download to test the download. You should be able to see a File Download dialog box appear on your screen.
If you have added the above request handler to your remote server, then replace localhost above, with your domain name or IP address.
Also read:
How to Fix Permission Denied Error in Django
How to Install mode_set_headers in NGINX
How to Get Data from URL in NodeJS
How to Setup Apache Virtual Hosts in Windows
How to Log POST Data in NGINX
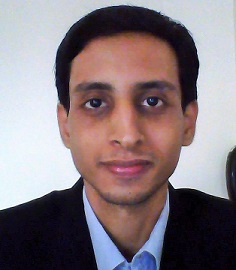
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.