NodeJS is a powerful javascript-based web development framework that allows you to build web applications and websites. Very often you may need to read data from requested URL’s querystring, in your applications. In this article, we will look at how to get data from URL in NodeJS.
How to Get Data from URL in NodeJS
Here are the steps to get data from URL in NodeJS. We will use ExpressJS for this purpose. If you have not installed ExpressJS you can do so with the following command.
$ npm install expressjs
1. Create NodeJS Server
Create app.js to be run as NodeJS server.
$ sudo vi app.js
Add the following lines to it.
var express = require('express'); var app = express();
The above lines will import ExpressJS. Next add the following lines to parse request body properly, dependin on ExpressJS version on your system.
ExpressJS >=4.16
app.use(express.bodyParser());
ExpressJS < 4.16
const bodyParser = require("body-parser");
app.use(bodyParser);
Next, add the following line to run server on port 80.
app.listen(80);
2. Add a Request Handler
Let us say you want to get data from URL http://127.0.0.1/test. For our example, we will pass id parameter in our URL http://127.0.0.1/test?id=45. In such case, add the following GET request handler in app.js.
app.get('/test', function(request, response) {
var id = request.query.id; // $_GET["id"]
console.log('id:'+id);
response.writeHead(200, {'Content-Type': 'text/html'})
response.send(id)
})
In the above code, we use request.query object to access our GET variables. If your request object is something else, like req, then you need to use req.query. In this object, you can access URL parameters’ values by simply using their name. In our example, we acces id parameter’s value using request.query.id.
We further print this value in console and also return it in our response.
If you want to read multiple parameters you can read them separately using their parameter names in request.query object. Here is an example, where we capture 2 parameters id and name in our URL http://127.0.0.1/test?id=45&name=abc
app.get('/test', function(request, response) {var id = request.query.id;
var name = request.query.name;
console.log('id:'+id); response.writeHead(200, {'Content-Type': 'text/html'}) response.send(id) })
Save and close the file.
3. Start NodeJS server
Start nodejs server with the following command.
$ sudo node app.js
Open browser and visit your URL http://127.0.0.1/test?id=45. In your server console you will see the id value of 45 displayed. You will also see it in your server response.
You can also run this server on your domain (e.g mysite.com) to capture get data from URL.
Also read:
How to Read POST data in NodeJS
NGINX Location Block Precedence
How to Fix Errno 13 Permission Denied in Apache
How to Define & Use Variables in Apache
NGINX Protect Static Files with Authentication
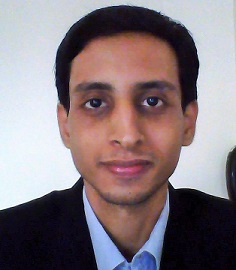
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.