Sometimes you may need to read command line arguments in NodeJS script. You may need to parse them and take different actions depending on their value. They allow you to build a customized NodeJS server that offers versatile features. In this article, we will look at how to process command line argument in NodeJS.
How to Read Command Line Arguments in NodeJS
Here are the steps to parse command line argument in NodeJS.
1. Create NodeJS file
Open terminal and run the following command to create an empty NodeJS file.
$ sudo vi arg.js
2. Parse Command Line Arguments
All command-line arguments in NodeJS are stored in a global variable process.argv as an array. So add the following line your NodeJS file to store it in a variable.
var args=process.argv;
Now you can access all arguments at one go by directly referring to your variable, as shown below
console.log(args);
Let us say you have the above code in arg.js that simply parses and displays command line arguments.
var args=process.argv; console.log(args);
If you run the above code as shown, you will see the command line arguments listed as an array.
$ node arg.js 1 2 3 ['node','/home/arg.js','1','2','3']
As you can see above, process.argv is an array of strings. The first item of this array (index=0) is node command, the second item(index=1) is the full path to NodeJS file. Your command line arguments begin from item with index 2. Here is an example to print first command line argument.
console.log(args[2]);
Here is an example that takes two command line arguments and displays their sum
var args=process.argv; console.log(parseInt(args[2])+parseInt(args[3]));
That’s it. In this article, we have learnt how to parse command line arguments in NodeJS. We have seen that they are available as an array of strings, with the first 2 items being node command and full file path to the NodeJS script file.
Also read:
How to Generate & Verify MD5 Hash of File in Linux
How to Install NVM on Mac using Brew
Shell Script to Start & Restart Tomcat Server
Shell Script to Backup MySQL Database
Htaccess Redirect If URL Contains String/Word
Related posts:
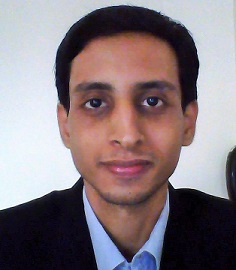
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.