Shell scripts allow you to automate many tasks and processes in Linux. They allow you to pass arguments in different ways. Sometimes you may need to read command line arguments in shell script. In this article, we will look at how to parse command line arguments in Bash. You can use the same method to read command line arguments in almost every shell.
How to Parse Command Line Arguments in Bash
Shell scripts allow you to parse command line arguments in 4 different ways. In this article, we will look at each of them one by one.
1. Positional Parameters
When you pass one or more parameters to shell script, it automatically assigns them variables to them, depending on their position. For example, the first argument can be accessed using $1 variable, the second variable via $2, and so on.
Here is an example to refer to command line arguments using bash. Let us say you have the following shell script test.sh with the following code
#!/bin/sh echo "First argument" $1 echo "Second Argument" $2
In the above code, we use $1 to access first argument value immediately after the command, and $2 to access second argument value. Now let us run the above script with 2 input parameters.
$ test.sh 'John' 'Doe'
You will see the following output
First argument John Second argument Doe
2. Using Flags
You can also define specific flags to pass each argument in shell script, just as you use options with Linux commands. In this case also, you need to use hyphen before each flag, and mention the argument value after the flag. Let us look at the following example that takes two arguments using flags f & s. We will modify the above shell script to use flag-based arguments instead of positional parameters.
while getopts f:s: flag do case "${flag}" in f) first=${OPTARG};; s) second=${OPTARG};; esac done echo "First: $first"; echo "Second: $second";
In the above code, we have defined two flags f and s for first and second argument respectively, using getopts. Now let us run them using flag-based parameters.
$ test.sh -f John -s Doe
Here is the output you will see.
First: John Second: Doe
3. Using Loop
Shell scripts also provide a readymade parameter $@ that stores an array of all input parameters. This is very useful if you have an unknown or variable number of parameters for your shell script. You can use this in a for loop, we can iterate over input and process it as per our requirement.
Let us look at an example. Let us modify the above shell script to loop through the input parameter and display them one by one.
#!/bin/sh for data in "$@" do echo "$data"; done
Let us run the shell script with the following command.
$ test.sh 1 2 3 4 5
Here is the output you will see.
1 2 3 4 5
4. Shift Operator
Shift operator allows you to shift the positions of input parameter by n positions, as per your requirement. If you do not specify n, it will shift the positional parameters by 1.
For example, by default, $1 refers to the first argument. But if you call it after “shift 2” command, then $1 will refer to the third argument.
Let us modify the above shell script to use shift command as shown below. $# contains the number of arguments used in shell script call.
#!/bin/sh i=1; j=$#; while [ $i -le $j ] do echo "$i: $1"; i=$((i + 1)); shift 1; done
Let’s run the above shell script as shown below
$ test.sh John Tim Tom
You will see the following output.
1: John 2: Tim 3: Tom
That’s it. In this article, we have looked at different ways to read command line arguments in shell script. Positional parameters can be used with you know the position of each parameter. In this case, order of parameters is important. With flags, you can input arguments in any order. Loop construct is useful when the number of input parameters is unknown or variable. Shift operator allows you to shift position of arguments based on your requirement.
Also read:
How to Install Varnish on CentOS for NGINX
How to Convert JPG to PDF in Linux
How to Install & Configure ElasticSearch in Linux
How to Use Strace Command in Linux
Top SFTP Command Examples
Related posts:
How to Read Variable from File in Shell Script
How to Compare Two Directories in Linux
How to Password Protect Folders in Linux
How to Pass Variable in cURL Command
How to Format USB Drives in Linux
How to Add Header in CSV File Using Shell Script
How To Run Multiple cURL Requests in Parallel
Sftp script to transfer files in Linux with password
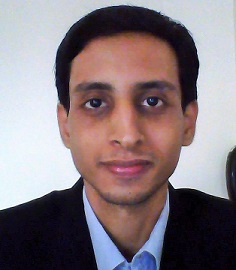
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.