NodeJS allows you to install & configure SSL certificates to enable HTTPS protocol support. HTTPS connections protect your websites from malicious attackers by encrypting the traffic between client browsers & your server. In this article, we will look at how to setup SSL/HTTPS in NodeJS server.
How to Setup SSL/HTTPS in NodeJS Server
Here are the steps to setup SSL/HTTPS in NodeJS server.
1. Download SSL certificates
The first step is to purchase & download SSL certificates from a third-party certificate authority like Symantec, RapiSSL, Comodo, GeoTrust, etc.
Once you have purchased the certificate and provided verification, the certificate authority will send SSL certificate files via email. It generally includes SSL certificate, Intermediate & Root certificate and CA bundle.
2. Create NodeJS server
Open terminal and run the following command to create a NodeJS server.
$ sudo vi server.js
Add the following lines in it. We use fs node module that allows NodeJS to read your certificate files.
var https = require('https'); var fs = require('fs'); var options = { key: fs.readFileSync("/path/to/private.key"), cert: fs.readFileSync("/path/to/your_domain_name.crt"), ca: [ fs.readFileSync('path/to/CA_root.crt'), fs.readFileSync('path/to/ca_bundle_certificate.crt') ] };
In the above code, we import http module to create server and fs module to work with SSL certificate files. We also create an options object that contains file paths to our certificate, private key, and ca bundle.
Here are the parameters defined in options object.
- path/to/private.key – full path of private key file
- path/to/your_domain_name.crt – full path of SSL Certificate file
- path/to/CA_root.crt – full path of CA root certificate file
- path/to/ca_bundle_certificate – full path of CA bundle file
Next add the following lines.
https.createServer(options, function (req, res) { res.writeHead(200); res.end("Welcome to Node.js"); }).listen(443)
The above code basically creates an HTTPS server running on port 443. We provide options variable defined above as an input to the server. This server will output “Welcome to Node.JS” message when you access it via HTTPS URL.
3. Start NodeJS Server
Run the following command to start your NodeJS HTTPS server.
$ sudo node start server.js
Open browser and visit https://your_domain and you should be able to “Welcome to Node.JS” response and the HTTPS protocol in address bar indicating that SSL is correctly setup on your website.
Also read :
How to Append File in NodeJS
How to Run NodeJS App in Background
How to Fix EADDRINUSE Error in NodeJS
How to Prevent Direct Access to PHP file
How to Use ES6 Import in NodeJS
Related posts:
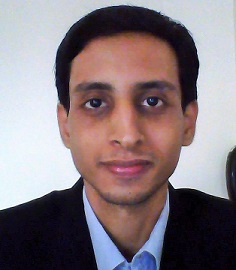
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.