JavaScript allows you to create and manipulate DOM elements. Sometimes you may need to provide a scroll to bottom feature on your website, especially if it is a blog which publishes long-form content. In this article, we will learn how to add scroll to bottom of div. There are several ways to do this, and you can use the same method to even scroll to the bottom of page.
How to Scroll to Bottom of Div
Let us say you have the following div.
<div id="myDiv"> ... </div>
1. Using JavaScript
You can easily scroll to the bottom of your div using the following code.
var objDiv = document.getElementById("myDiv"); objDiv.scrollTop = objDiv.scrollHeight;
You can add this code in the event handler of a button on your page, so that it is triggered when button is clicked.
<button onclick="myFunction()">Click me</button> <div id="myDiv"> ... </div> <script> myFunction(){ var objDiv = document.getElementById("myDiv"); objDiv.scrollTop = objDiv.scrollHeight; } </script>
2. Using jQuery
You can also use jQuery’s scrollTop() function for this purpose.
$("#myDiv").scrollTop($("#myDiv")[0].scrollHeight);
You can add this as an event handler on clicking of a button, or as per your requirement.
<button id='myButton'>Click me</button> <div id="myDiv"> ... </div> <script> $('#myButton').click(function(e){ $("#myDiv").scrollTop($("#myDiv")[0].scrollHeight); }); </script>
In this article, we have learnt how to scroll to bottom of div. If you want to scroll to the bottom of the page, you can simply change the id of div to that of the body. Also, we have called these functions on the click of a button, but you can also execute them on page load to automatically scroll down to the bottom of your div or page, as per your requirement.
Also read:
How to Remove Element from JS Array
How to Check if Variable is Array in JS
How to get Highlighted/Selected Text in JS
How to Call JS Function in IFrame from Parent Page
How to Prevent Page Refresh on Form Submit
Related posts:
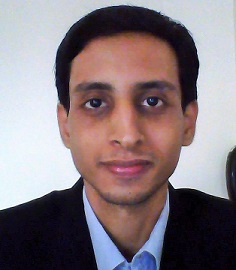
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.