Typically, we need to split a string with using single delimiters in python or any other programming language. But sometimes you may need to split a string with multiple delimiters in python. It can be tedious to manually split the string one by one using a single delimiter, iteratively. In this article, we will learn how to split string with multiple delimiters in Python, in a single step.
How to Split String With Multiple Delimiters in Python
Let us say you have the following string in python.
a = "Hello World, Good Morning"
Let us say you want to split the above string by space as well as comma characters into ‘Hello’, ‘World’, ‘Good’, ‘Morning’. You can easily do this using regular expressions in Python. It provides a built in library function re.split() function that allows you to easily split a string by multiple delimiters.
import re re.split(' |, ', a)
In the above function, we provide two arguments. The first argument is a sequence of delimiters to split the string. These delimiters need to be separated by OR (|) operator. In our case, we have mentioned space, comma and space as delimiters. The second argument is the string to be split.
You can also use the regular expression shortcut \s for white space.
import re re.split('\s|, ', a)
In both cases, you will get the following output.
['Hello', 'World', 'Good', 'Morning']
Alternatively, you can call replace() function on the string to replace all delimiters by single delimiter and then call split function to split it.
a.sub(r'\s|, ',',') a.split(',')
In this article, we have learnt how to split string with multiple delimiters in python using re.split() function. It is very powerful and concise. It is a lot easier than iteratively splitting string for each delimiter, or replacing each delimiter with the same delimiter and then calling split function to split the string using the single delimiter.
Also read:
How to Clone Array in JavaScript
How to Check MySQL Storage Engine Type
How to Use LIKE Operator in SQL
How to Increase Import File Size in PHPMyAdmin
How to Add Column After Another Column in MySQL
Related posts:
How to Get Difference of Two Dictionaries in Python
How to Remove All Occurrences of Value from List in Python
How to Check if Substring is in List of Strings
How to Compare Dictionary in Python
How to Convert Webpage into PDF using Python
How to Randomly Select Item from List in Python
How to Repeat String N Times in Python
How to Flatten List of Lists in Python
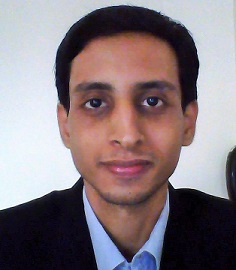
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.