Sometimes you may need to use Boolean Variables in shell script. In this article, we will learn how to declare, set and evaluate Boolean variables in Shell script. We will learn how to set variable to Boolean value and also how to check if a variable is Boolean.
How to Use Boolean Variables in Shell Script
In shell scripts, you don’t need to declare variables. You can directly assign their values and they will be evaluated at runtime. Here is a simple syntax to set variable to Boolean Values.
var = boolean_value
Here are two examples to set variable abc to true and false.
abc = true abc = false
Next, we will learn how to evaluate a Boolean variable’s value.
abc=true if [ "$abc" = true ] ; then echo 'abc is true' fi
In the above example, we assign variable’s value to True. We use if condition to check if variable is True. You might be tempted to use the following syntax to check if a shell variable is true, as is done in other programming languages.
abc=true if $abc ; then echo 'abc is true' fi
Avoid the above way of checking Boolean variable in shell script. This is because bash shell has a built true value for each assigned variable, irrespective of its value. For example, the following statements will also cause the above condition to evaluate to true.
abc='' if $abc ; then echo 'abc is true' fi abc='xyz' if $abc ; then echo 'abc is true' fi abc=some command if $abc ; then echo 'abc is true' fi
In each of the above 3 examples, the if condition evaluates to true value and executes the nested script. This is because, in such cases, bash only checks if the shell variable is set or not, and not its assigned value. So it can be insecure where even a proper command might end up getting evaluated, as shown in example 3.
Here are some examples of checking if a variable is true, that are all equivalent.
abc=true if [ "$abc" = true ]; then if [ "$abc" = "true" ]; then if [[ "$abc" = true ]]; then if [[ "$abc" = "true" ]]; then if [[ "$abc" == true ]]; then if [[ "$abc" == "true" ]]; then if test "$abc" = true; then if test "$abc" = "true"; then
In this article, we have learnt how to set shell variable to Boolean value as well as evaluate it.
Also read:
How to Concatenate String Variables in Shell Script
How to Iterate Over Arguments in Shell Script
Bash Script That Takes Optional Arguments
How to Test if Variable is Number in Shell Script
How to Check if Variable is Number in Python
Related posts:
How to Fix Permission Denied Error While Running Shell Script
How to Reset Password in Ubuntu
How to Create Symbolic links in Linux
How to Find All Symlinks to File/Directory
How To Install Pip in Ubuntu Linux
How to Unban IP With Fail2ban
How to Disconnect User from SSH Connection
How to mkdir Only if Directory Does Not Exist
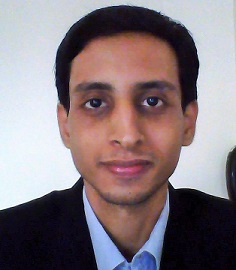
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.