Sometimes you may need to ask user for input in your shell script. There are several ways to prompt user for input in shell script, using read command. In this article, we will learn about them one by one. You can use these commands on all shells in every Linux distribution.
How to Prompt for User Input in Shell Script
Linux shells provide read command that allows you to read one or more inputs from users in your shell script. Here is its syntax.
read <variable_name>
After read command, you need to mention the variable in which you want to store the user input.
We will look at some common use cases for read command.
1. Read Single Input
In this cases, we simply prompt user for single input and store it in a single variable.
#/home/test.sh #!/bin/sh echo "enter name:" read name echo "you entered $name"
When you run the above script you will see the following prompt. On entering an input, the script will store the user input in $name variable, and echo it back to the screen.
$ /home/test.sh enter name:fedingo you entered fedingo
2. Read Multiple Inputs
You can also use read command to prompt for multiple inputs. In this case, you need to specify the different variable names one after the other in read command.
read variable1 variable2 variable3 ...
In this case, the user needs to input the different variable values one after the other in space-separated format and hit enter key after you type the value for last variable, to submit the input.
#/home/test.sh #!/bin/sh echo "enter first name, last name and age:" read first_name last_name age echo "you entered $first_name, $last_name, $age
When you run the above script, it will prompt you to enter 3 values – first name, last name and age. When you enter 3 values in a space-separated format, it will store them in the 3 shell variables $first_name, $last_name and $age respectively, and echo these values back to screen.
$ /home/test.sh enter first name, last name and age:john doe 42 you entered john, doe, 42
If you don’t know how many inputs to expect from user, or want to accept a variable number of inputs, then you can also use an array to store user input values accepted using read command. In this case, you need to add -a option after read command, to be able to store input values in array. Here is an example to store the first name, last name and age values in details array.
#/home/test.sh #!/bin/sh echo "enter first_name, last_name and age" read -a details echo "you entered ${details[0]},${details[1]},$details{details[2]}
When you run the above script, you will be prompted for first name, last name and age. When you enter these values, they will be stored in details array.
$ /home/test.sh enter first name, last name, age:john doe 42 you entered john, doe, 42
Since you are using an array, user can enter any number of inputs and your shell script will capture them properly. It is a great way if you want to accept a list of values such as names, places, etc.
3. Accept Input Without Variable
If you don’t mention variable name, then shell script will store it in $REPLY system variable. Here is an example
#/home/test.sh #!/bin/sh echo "enter name" read echo "you entered $REPLY"
When you run the above command, you will be prompted for input. The string you enter will be stored in $REPLY variable, and echoed back to your screen.
$ /home/test.sh enter name: fedingo you entered fedingo
4. Hide User Input
Sometimes you may want to hide the user input when they are typing. For example, if you want to prompt user for sensitive information like passwords, you will need to hide it. You can do so using -sp option with read command, where -s stands for silent mode and -p stands for prompt. In silent mode, the shell will not display what user types for input. Here is an example.
#/home/test.sh #!/bin/sh echo "enter password:" read -sp password echo "you entered $password"
When you run the above command, you will be prompted for password. What you type will not be visible until you hit enter key. At that time, the script will echo back the full input you entered.
$ /home/test.sh enter password: you entered 1234
In this article, we have learnt several ways to accept user input in shell script using read command, which is quite versatile and useful.
Also read:
How to Uninstall SQL Server in Ubuntu
How to Install or Upgrade Unsupported Packages in Ubuntu
Shell Script to Check if Script is Already Running
How to Check If Input Arguments Exist in Shell Script
How to Check if Variable is Empty or Not in Shell Script
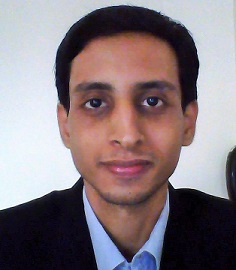
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.