When you run shell script it is important to ensure that only a single instance of your script is running. If you run the command to start your shell script when another instance of it is already running, it can lead to unpredictable result. So before you run your shell script it is important to check if it is already running. Here is how to check if script is already running on your system.
Shell Script to Check if Script is Already Running
We will look at different ways to check if script is already running. Let us say you want to check if a shell script test.sh is running or not.
1. From Shell
In this case, we will check from terminal shell if a given script is running.
if pidof -x "test.sh" >/dev/null; then echo "Process already running" fi
Every running process has a PID. We use pidof command to determine the PID of our shell script. If the PID exists, it means the shell script is already running. In such cases, the above script will display a message saying that the process is already running.
2. From Within Script
If you want to check whether the script is running from within the script, you can embed the above commands within the shell script. Here is an example. Add the following code in test.sh.
#!/bin/bash for pid in $(pidof -x test.sh); do if [ $pid != $$ ]; then echo "[$(date)] : abc.sh : Process is already running with PID $pid" exit 1 fi done
Now when you run the script it will automatically check for all instances of the script that are running and print their PIDs. If there is no other instance running, then the above code will print only 1 PID, that of the script. If there are other instances running, then it will print more than 1 PID. That is why we use a for loop to display all PIDs of the script instances.
3. Create PID file
You can also create PID file to determine if a shell script is running. In this case, we will create a PID file when we run the script, and delete the file when we exit the script.
#!/bin/bash # test.sh mypidfile=/var/run/test.sh.pid # Add check for existence of mypidfile if [ -f $mypidfile ]; then echo "script is already running" exit fi # Ensure PID file is removed on program exit. trap "rm -f -- '$mypidfile'" EXIT # Create a file with current PID to indicate that process is running. echo $$ > "$mypidfile" ...
In the above code, we define the path to PID file, and check if the file exists. If so, the program exits with a message saying that the script is already running. Else, it creates a PID file as long as the script is running. When the script exits then the PID file is automatically deleted, using trap & rm commands.
In this article, we have learnt different ways to check if another instance of shell script is running on your system. If you find that your shell script is already running and has become non-responsive, then you can use the kill command to kill the process, and then start a new instance of it.
Also read:
How to Check if Input Argument Exists in Shell Script
How to Check if Variable is Empty or Not in Shell Script
How to Check if File Exists in Shell Script
How to Check if Directory Exists in Shell Script
How to Check if Directory Exists in Python
Related posts:
How to Get User Input in Shell Script
How to Edit Hex Files in Linux
How to Install Supervisor in RHEL/CentOS/Fedora
Sed Command to Replace String in File
How to Record & Replay Terminal Session in Linux
How to Use Journalctl Command in Linux
How to Run Same Command Multiple Times in Linux
How to Disable Swap in Linux
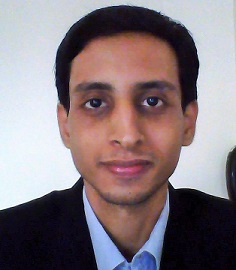
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.