Python allows you to work with files and store its data into different data structures such as lists, dictionaries, tuples, etc. In this article, we will learn how to read file line by line and store each line as an item in list. In this article, we will learn how to read file line by line into python list.
How to Read File Line by Line Into Python List
There are several ways to read files line by line and store each line as a list item in python.
Here is the simple command to open file and load it completely into memory as a list named lines. readlines() function returns a list with each line as a separate item.
with open(filepath) as file: lines = file.readlines()
Here is an example to load contents of /home/ubuntu/data.txt into memory.
with open('/home/ubuntu/data.txt') as file: lines = file.readlines()
If you want to remove whitespace and newline characters from end of each line in the file, modify the above code as shown below.
with open(filepath) as file: lines = [line.rstrip() for line in file]
In the above use case, if you want to remove only newline characters but retain whitespace characters at the end of each line, you can modify the above code as shown.
with open(filepath) as f: lines = [line.rstrip('\n') for line in f]
If you are working with large files, the above code may overwhelm your system, since it loads the entire file into memory. In such cases, it is advisable to read and process the file line by line.
file_list=[] with open(filepath) as file: for line in file: file_list.append(line.rstrip())
If you are using python 3.8+, you can also use while loop as shown below.
file_list=[] with open(filepath) as file: while line := file.readline(): file_list.append(line.rstrip())
In this article, we have learnt how to read files line by line in python and append each line to a list. You can use it as a part of a bigger function or module, or modify it as per your requirement.
Also read:
How to Backup WordPress to GitHub
How to Backup WordPress to Google Drive
How to Print Without Newline or Whitespace in python
How to Convert String Representation of List to List
How to Set Current Working Directory to Shell Script Directory
Related posts:
How to Read User Input in Python
How to Split Python List into N Sublists
How to Uninstall Django in Ubuntu
How to Execute Stored Procedure in Python
How to Check Python Package Dependencies
How to Reverse/Invert Dictionary Mapping in Python
How to Setup Uwsgi with NGINX for Python
How to Compare Dictionary in Python
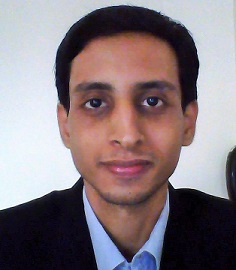
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.