Sometimes you may need to get user input in shell script. In this article, we will look at the different ways to get user input using read command in shell script.
How to Get User Input in Shell Script
You can use read command to accept user input in your shell script. Here is its syntax
read <variable_name>
We will look at different use cases to use read command.
1. Get single input
You can simply mention your variable name after read command to read and store user input in that variable. Here is an example of shell script
#/home/test.sh #!/bin/sh echo "enter name:" read name echo "you entered $name"
In the above code, we use read command to read a single input and store it in variable name.
$ /home/test.sh enter name:fedingo you entered fedingo
2. Get Multiple User Input
You can also read multiple variables by mentioning them one after the other after read command. Here is its syntax.
read variable1 variable2 variable3
Here is an example where we accept multiple variables first_name, last_name and age as user input.
#/home/test.sh #!/bin/sh echo "enter first_name, last_name and age:" read first_name last_name age echo "you entered $first_name, $last_name, $age
You need to enter the different user inputs in a space-separated format. When you have typed all input variable, press enter to pass them to shell script.
$ /home/test.sh enter name:john doe 42 you entered john, doe, 42
You may also use an array to store multiple inputs
#/home/test.sh #!/bin/sh echo "enter first_name, last_name and age" read -a details echo "you entered ${details[0]},${details[1]},$details{details[2]}
In this case, type the user input values in a space separated manner, and press enter when you are ready to submit, just like above.
$ /home/test.sh enter first name, last name, age:john doe 42 you entered john, doe, 42
The main difference in this case is that since you are using an array, users can enter any number of input values and shell script will automatically store them in array elements.
3. Hide User Input
Sometimes you may need to hide user input as they are typing. For example, if you are reading password, then you will need to hide user input but read it correctly. For this purpose, use read command with -sp option. In this case, -s stands for silent mode and -p stands for command prompt. Here is an example
#/home/test.sh #!/bin/sh echo "enter password:" read -sp password echo "you entered $password"
In this case, your users will see the prompt, but when they enter input, it will not be visible. Only when they press enter key it will be submitted.
$ /home/test.sh enter password: you entered 1234
4. Get User input without variable
If you don’t use variable in read statement, then shell script will store user input in REPLY system variable.
#/home/test.sh #!/bin/sh echo "enter name" read echo "you entered $REPLY"
In the above case, you can echo the value of $REPLY variable.
$ /home/test.sh enter name: fedingo you entered fedingo
Read is a very versatile command to process input in shell script. In this article, we have looked at different use cases to read user input in shell script.
Also read:
Shell Script to Get CPU & Memory Utilization
How to Check SSD Health in Linux
How to Set Default Gateway in Linux
How to Touch All Files in Directory
How to Exit For Loop in Shell Script
Related posts:
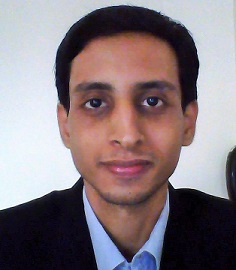
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.