Shell Scripts allow you to loop through commands using for and while statements. But sometimes you may need to exit loops in between before completion. For this purpose, Linux provides you break statement. In this article, we will look at how to come out of for loop in shell script. You can use these steps for all Linux distributions since break statement is available in all Linux systems.
How to Exit For Loop in Shell Script
We will use break statement to come out of for loop (and while loop) in shell script. Here is the syntax of break statement.
break OR break N
We will look at three common use cases to exit loops
- Using for statement
- Using while statement
- Using nested for statement
Exit For Loop
Here is a simple example (break.sh) to exit for loop using break statement. In the following statement, the for loop breaks if the variable i‘s value is equal to the one received using command line argument $1. Otherwise it continues with the loop.
#!/bin/sh i=1 for $i < 10 do if [ $i -eq $1 ] then echo "found matching value" break fi done if [ $i -eq 10 ] then echo "match not found" fi
Save and close the file. You can run it with the following commands. We pass command line argument 3 to make it break out of loop when i=3.
$ sudo chmod +x break.sh $ ./break.sh 3
Exit While Loop
Here is the above example using while loop and break statement. In the following statement, the while loop breaks if the variable i‘s value is equal to the one received using command line argument $1. Otherwise it continues with the loop.
#!/bin/sh i=1 while $i < 10: do if [ $i -eq $1 ] then echo "found matching value" break fi done if [ $i -eq 10 ] then echo "match not found" fi
Save and close the file. You can run it with the following commands. We pass command line argument 3 to make it break out of loop when i=4.
$ sudo chmod +x break.sh $ ./break.sh 4
Using Nested Loops
Please note, when you use break statement alone, then it will exit only the immediate loop. If you are working with nested loops and want to exist all loops at once, you need to use ‘break N’ statement where N is the level of nesting.
For example, if you have one loop nested inside another, and you want to exit both loops then use break 2 statement, as shown below. It simply breaks out of both the loops if value of i matches that of command line argument.
#!/bin/sh i=1 for [ $1 < 10] do while true do [ $i -eq $1 ] && break 2 done done
Save and close the file. You can run it with the following commands.
$ sudo chmod +x break.sh $ ./break.sh 5
That’s it. In this article, we have learnt how to exit for loop, while loop and even nested loops in Linux.
Also read:
How to Set Default Python Version in Linux
How to Install MediaWiki with NGINX in Linux
How to Send Email with Attachment in Linux
How to Remove (Delete) Symbolic Links in Linux
How to Install Zoom in Ubuntu
Related posts:
How to Run MySQL Query from Command Line
Shell Script to Automate SSH Login
How to Find & Kill Zombie Processes in Linux
How to Sort Files by Size in Linux
How to Use Wget to Download File Via Proxy
Tar Directory Exclude Files & Folders
How to Grep Log File Within Specific Time Period in Linux
How to Limit User Commands in Linux
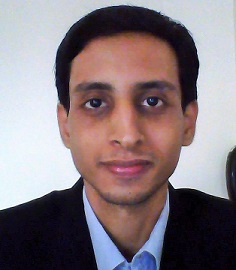
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.