JavaScript DOM structure allows you to nest HTML elements one inside the other or one after another providing an extremely flexible layout that allows you to easily access any element quickly. Often you may need to remove all child elements of DOM node in JavaScript. In this article, we will learn how to remove all child elements of DOM Node in JS. There are several ways to do this and we will look at them one by one.
How to Remove All Child Elements of DOM Node in JS
Let us say you have the following HTML.
<div id="myDiv"> <span>hello</span> <div>world</div> </div>
Let us say you want to remove all child elements of div with id=’myDiv’ so that it looks like the following.
<div id="myDiv"> </div>
Here are some of the ways to remove its child nodes.
1. Clearing InnerHTML
First we get a hold of our div using getElementById() function.
myNode = document.getElementById("myDiv");
Every DOM node supports innerHTML property which contains HTML of all the children and other HTML code inside the given node. You can empty it by setting innerHTML to ” as shown below. This will remove all its children.
myNode.innerHTML = '';
Please note, this will invoke browser’s HTML parser and may be slow if you have too many children nodes.
2. Clearing TextContent
Each DOM node also supports TextContent property that can also be emptied by setting to null string. In fact, it is faster than setting innerHTML to ” since it does not invoke web browser’s HTML parser.
var myNode = document.getElementById("myDiv"); myNode.textContent = '';
3. Using Loop to Remove LastChild
You can also loop through the children DOM elements to remove each of them. In the following code, we loop through the child elements of div by looking for lastChild element and remove them one by one.
myNode = document.getElementById("myDiv"); while (myNode.lastChild) { myNode.removeChild(myNode.lastChild); } OR myNode = document.getElementById("myDiv") while (myNode.lastChild) { myNode.lastChild.remove() }
The first code above calls removeChild() function of parent node while the second code calls remove() function on the child node itself.
You can also do the same thing using firstChild because in some browsers it is faster to remove the firstChild while in others it is faster to remove the lastChild.
myNode = document.getElementById("myDiv"); while (myNode.firstChild) { myNode.removeChild(myNode.firstChild); } OR myNode = document.getElementById("myDiv") while (myNode.firstChild) { myNode.firstChild.remove() }
This approach is slower than the first two methods since you need to loop through the DOM elements but it is more powerful because it allows you to selectively remove only certain child elements if you want to and also retain non-element HTML such as plain texts, comments, etc.
4. Using Loop to Remove lastElementChild
Similarly, you can also use loops to iterate through child DOM elements and remove lastElementChild one by one.
var myNode = document.getElementById("myDiv"); while (myNode.lastElementChild) { myNode.removeChild(myNode.lastElementChild); } OR myNode = document.getElementById("myDiv") while (myNode.lastElementChild) { myNode.lastElementChild.remove() }
You can also use firstElementChild instead of lastElementChild.
This uses the same approach as previous one. The main difference between using lastChild and lastElementChild is that lastChild returns element node, text node and comment node whereas lastElementChild returns only element node.
In this article, we have learnt several ways to remove child nodes of DOM node. You can use any approach depending on your requirements.
Also read:
How to Check If Variable Exists or is Defined in JS
How to Convert UTC Datetime to Local Datetime in JS
How to Use Variable Number of Arguments in JS Function
How to Add 1 Day to Current Date in JavaScript
How to Change One Character in Python String
Related posts:
How to Check if JS Object Has Property
How to Scroll to Bottom of Div
How to Convert Array to Object in JavaScript
How to Get Unique Values from Array in JavaScript
How to Auto Resize IFrame Based on Content
How to Get Client IP Address Using JavaScript
How to Use Multiple jQuery Versions on Same Page
How to Call JS Function in IFrame from Parent Page
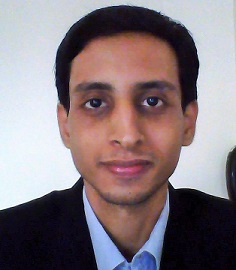
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.