Regular Expression (Regex) is a sequence of characters use for defining a pattern. You can use it for searching, pattern matching and string replacement. It is often used for input validation to check if a given user input conforms to a specific pattern. So many times you may need to check if string matches regular expression and raise an exception if it isn’t so. In this article, we will learn how to check if string matches regular expression.
How to Check if String Matches Regular Expression
We will use inbuilt re library to work with regex and strings in python. There are two aspects to pattern matching in Python. The first part is to check if your regex itself is valid or not. The second part is to check whether a given string matches your regex or not. The first part is optional and often skipped by developers but it is a best practice to do it.
1. Check Validity of Regex String
Here is a simple code to check the validity of a given regular expression. We will look at it in detail.
import re # pattern is a string containing the regex pattern pattern = r"[.*" try: re.compile(pattern) except re.error: print("Non valid regex pattern") exit()
Let us look at the above code in detail. First we import the re library to process the regular expressions. We define our regex string in pattern variable. We use re.compile() function to test the validity of our regular expression. If the regex is invalid, the re library will raise an re.error exception. So we enclose the re.compile() function in a try..except block. The except block is defined to catch this re.error type of exception and print that our regex is not valid, thereby exiting the code.
2. Check if String Matches Regex
In this case, we will learn how to check if a given string matches regex. Here is a sample code that first checks if a regex is valid or not, and then check if a given string matches the regex or not.
import re # pattern is a string containing the regex pattern pattern = r"[A-Za-z0-9]+" try: re.compile(pattern) # Prompts the user for input string test = input("Enter the string: ") # Checks whether the whole string matches the re.pattern or not if re.fullmatch(pat, test): print(f"'{test}' is an alphanumeric string!") else: print(f"'{test}' is NOT a alphanumeric string!") except re.error: print("Non valid regex pattern") exit()
In the above code, we first import re library as we did before. Then, within try block, we first define regex pattern for alphanumeric string, use re.compile() function to check if our regular expression is valid or not. Then we call input() function which basically prompts user for input string. Then we use re.fullmatch() function to test if user input is a valid alphanumeric string by checking it against our regex. If it matches the regex, fullmatch() function will return true, else it will return false. Accordingly, we display the message. As described before, we also use an except block to catch the re.error exception, in case our regex is not valid.
You can customize both these codes to check the validity of a regex, and check if a string matches your regex or not.
In this article, we have learnt how to check if a string matches regular expression in python.
Also read:
How to Modify XML File in Python
Plot Graph from CSV Data in Python
How to Encrypt Password in Python
How to Create Thumbnail in Python
How to Open Multiple Files in Vim
Related posts:
How to Concatenate Items in List Into String in Python
How to Randomly Select Item from List in Python
How to Convert Columns into Rows in Pandas
How to Copy List in Python
How to Convert CSV to Tab Delimited File in Python
How to Check Python Package Path
How to Import from Parent Folder in Python
How to Store JSON to File in Python
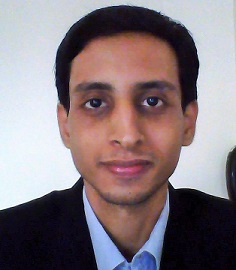
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.